Chapter 6
Modern CNN Architectures
"Architectures like ResNet and DenseNet have fundamentally changed how we think about deep learning. Implementing these models in Rust opens new possibilities for performance and scalability in AI." — Geoffrey Hinton
Chapter 6 of "Deep Learning via Rust" (DLVR) offers a detailed exploration of modern Convolutional Neural Networks (CNNs), tracing their evolution from foundational models to the advanced architectures shaping contemporary deep learning. The chapter introduces key networks such as VGG, ResNet, Inception, DenseNet, EfficientNet, MobileNet, and YOLO, emphasizing their innovations in addressing challenges like the vanishing gradient problem and the demand for scalable, efficient models. It delves into the principles and practical implementations of these architectures, highlighting features like residual connections, multi-scale feature extraction, dense connectivity, and efficient scaling strategies. Special attention is given to EfficientNet and MobileNet's neural architecture search (NAS) for optimizing depth, width, and resolution, as well as YOLO's real-time object detection capabilities. Through Rust-based implementations using libraries like tch-rs, readers gain hands-on experience in building, training, and fine-tuning these architectures, leveraging Rust’s performance for cutting-edge deep learning applications.
6.1. Introduction to Modern CNN Architectures
Convolutional Neural Networks (CNNs) have undergone significant evolution since their inception, progressing from simple architectures like LeNet to highly complex, powerful models such as ResNet, Inception, and EfficientNet. The primary focus of CNN evolution has been on increasing both the depth and complexity of networks to improve their ability to learn hierarchical, abstract features from data. Early CNNs like LeNet and AlexNet had only a few convolutional layers, limiting the extent of feature extraction possible. However, with advancements in computation and deep learning research, modern CNN architectures have grown deeper and wider, incorporating architectural innovations that allow them to tackle increasingly complex tasks across domains such as image classification, object detection, and segmentation.
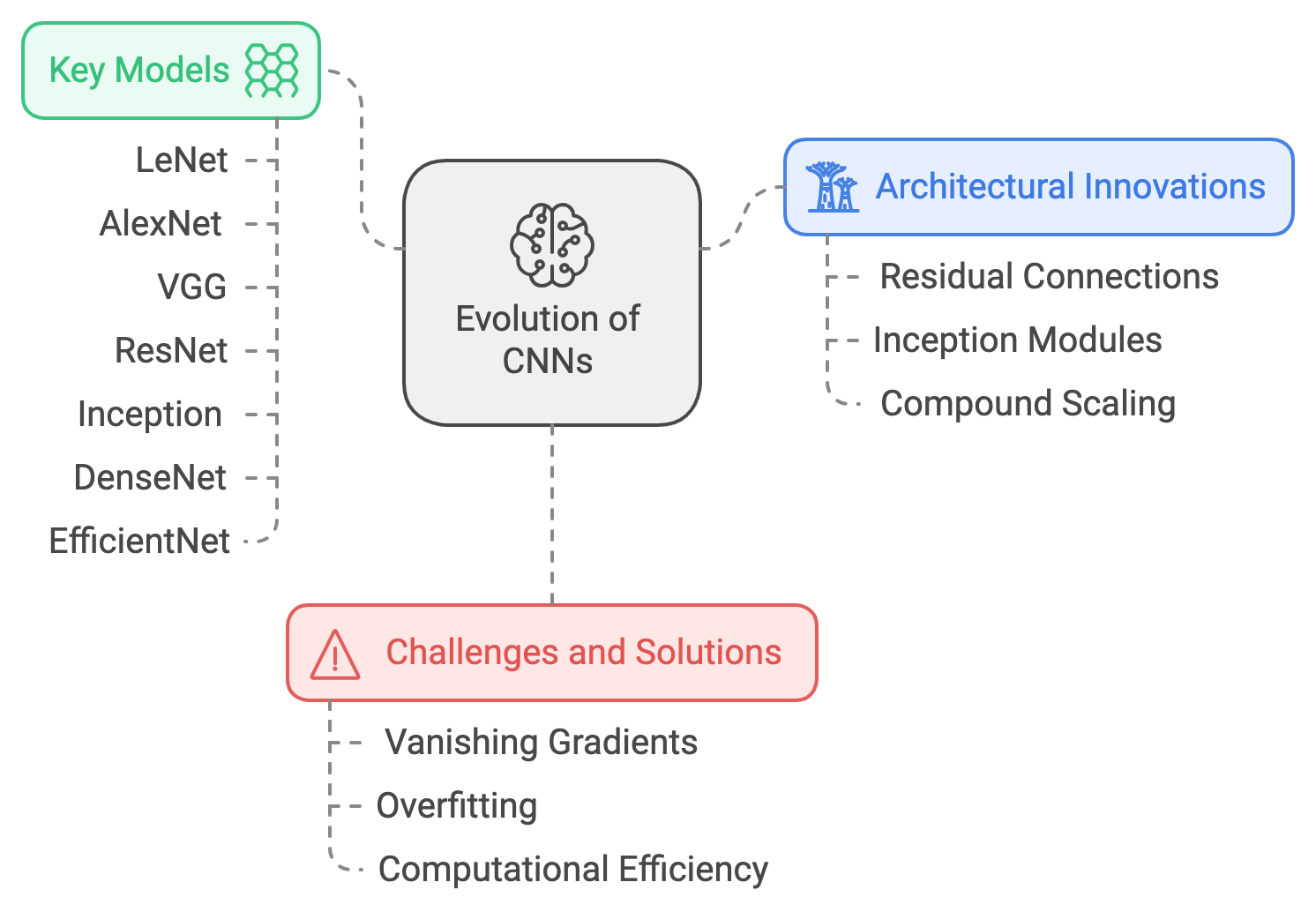
Figure 1: The modern evolution of CNNs.
One of the critical innovations driving the development of modern CNNs has been the ability to make networks deeper while mitigating the challenges associated with training deep networks. One such innovation is the use of residual connections, introduced in ResNet. Residual connections allow information to bypass several layers, thus addressing the vanishing gradient problem, which often plagues deep networks. Mathematically, residual connections allow the network to learn an identity mapping alongside the transformation learned by the layers, formalized as $y = f(x) + x$, where $f(x)$ represents the learned transformation and $x$ is the input passed directly to the output. This allows gradients to flow more effectively during backpropagation, enabling networks to have hundreds of layers without suffering from performance degradation.
Inception modules, introduced in the Inception architecture, provide another important innovation in CNN design by enabling multi-scale feature extraction. Instead of applying a single filter size at each layer, Inception modules apply multiple filters (such as 1x1, 3x3, and 5x5) in parallel, allowing the network to capture both fine and coarse features from the same input. This multi-scale approach is mathematically represented as $y = \text{Concat}(f_{1x1}(x), f_{3x3}(x), f_{5x5}(x))$, where the results of applying different filters are concatenated into a single output tensor. This architectural design allows the network to efficiently capture a diverse range of patterns, from small details to large structures.
Another major innovation in modern CNN architectures is the focus on parameter efficiency, as demonstrated in EfficientNet. The EfficientNet family of models introduces a method called compound scaling, which scales network width, depth, and input resolution in a balanced manner. By optimizing how networks grow across these dimensions, EfficientNet achieves high accuracy while maintaining computational efficiency. This innovation is particularly valuable in resource-constrained environments, such as mobile devices, where both performance and efficiency are critical.
Several of the most impactful modern CNN architectures highlight these innovations. VGG networks, for example, demonstrated the importance of depth by stacking small convolutional filters (3x3) and increasing the number of layers to improve representational capacity. Although VGG networks are computationally expensive due to their large number of parameters, they served as a critical step in understanding how deeper networks could improve performance. ResNet, on the other hand, fundamentally changed how deep networks are built by introducing residual connections, allowing networks with hundreds of layers to be trained effectively. In contrast to VGG, ResNet reduced the number of parameters while maintaining strong performance, making it a more efficient architecture.
The Inception network, with its unique multi-scale feature extraction, brought a new level of flexibility to CNNs. By applying filters of different sizes within the same layer and concatenating their outputs, the Inception architecture allows the model to learn features across various scales without increasing the computational cost dramatically. This makes Inception particularly well-suited for tasks where features of varying sizes need to be captured, such as object detection and scene understanding.
DenseNet further built upon the concept of skip connections by introducing dense connections, where each layer receives input from all preceding layers. This design maximizes feature reuse and improves the flow of gradients, enabling DenseNet to achieve high accuracy with fewer parameters than traditional architectures. Finally, EfficientNet exemplifies the trend toward balancing network complexity with computational efficiency. By introducing a systematic approach to scaling networks across depth, width, and resolution, EfficientNet achieves state-of-the-art performance while using fewer resources, making it an ideal architecture for deployment on edge devices and mobile platforms.
Despite their numerous benefits, training very deep CNNs presents challenges that have required novel solutions. One major challenge is the vanishing gradient problem, where gradients diminish as they propagate through the layers, making it difficult to update the earlier layers of the network. Innovations such as residual connections in ResNet and dense connections in DenseNet have helped solve this problem by creating direct paths for gradients to flow backward through the network, allowing for more effective training of deep models. Another challenge is overfitting, which becomes more pronounced in deeper networks due to the increased number of parameters. Techniques like dropout, data augmentation, and weight decay have been widely adopted to mitigate overfitting and improve generalization, particularly when training on smaller datasets. Additionally, computational efficiency becomes a concern as networks grow deeper and wider. EfficientNet’s compound scaling strategy addresses this issue by optimizing the growth of network dimensions in a balanced way, ensuring that resources are used efficiently without compromising performance.
In terms of practical implementation, Rust offers a powerful and efficient platform for building and deploying CNNs, particularly in performance-critical applications. The tch-rs
crate provides robust tools for defining and training modern CNN architectures in Rust. A typical implementation might involve setting up a ResNet model with residual connections or creating an Inception-like module for multi-scale feature extraction.
The following example shows how to implement a simple ResNet-inspired architecture in Rust using the tch-rs
library. The following implementation uses Rust's tch
library to define a ResNet-like architecture tailored for the CIFAR-10 dataset. It includes a base convolution layer, followed by three residual blocks with increasing feature maps, and concludes with a fully connected layer for classification. The code provides a compact and efficient implementation of ResNet principles for image recognition tasks.
[dependencies]
anyhow = "1.0"
tch = "0.12"
reqwest = { version = "0.11", features = ["blocking"] }
flate2 = "1.0"
tokio = { version = "1", features = ["full"] }
tar = "0.4.43"
use anyhow::{Result, Context};
use flate2::read::GzDecoder;
use reqwest;
use std::{fs, path::Path};
use tar::Archive;
use tch::{nn, nn::ModuleT, nn::OptimizerConfig, Device, Tensor, vision};
/// URL for the CIFAR-10 dataset
const CIFAR10_URL: &str = "https://www.cs.toronto.edu/~kriz/cifar-10-binary.tar.gz";
/// Function to download and extract the CIFAR-10 dataset.
async fn download_cifar10() -> Result<()> {
let dest = "data/cifar10";
if Path::new(dest).exists() {
println!("CIFAR-10 dataset already exists, skipping download.");
return Ok(());
}
fs::create_dir_all(dest).context("Failed to create data directory")?;
println!("Downloading CIFAR-10 dataset...");
let response = reqwest::get(CIFAR10_URL).await?;
let bytes = response.bytes().await?;
let tar_gz = GzDecoder::new(&bytes[..]);
let mut archive = Archive::new(tar_gz);
println!("Extracting CIFAR-10 dataset...");
archive.unpack("data")?;
println!("CIFAR-10 dataset downloaded and extracted successfully.");
Ok(())
}
#[tokio::main]
async fn main() -> Result<()> {
// Ensure the CIFAR-10 dataset is downloaded and extracted.
download_cifar10().await?;
// Run the ResNet model training.
run_resnet()
}
// Define the ResNet architecture.
#[derive(Debug)]
struct ResNet {
conv1: nn::Conv2D,
bn1: nn::BatchNorm,
layer1: ResidualBlock,
layer2: ResidualBlock,
layer3: ResidualBlock,
fc: nn::Linear,
}
impl ResNet {
fn new(vs: &nn::Path) -> ResNet {
let conv1 = nn::conv2d(vs, 3, 64, 3, Default::default());
let bn1 = nn::batch_norm2d(vs, 64, Default::default());
let layer1 = ResidualBlock::new(vs, 64, 64);
let layer2 = ResidualBlock::new(vs, 64, 128);
let layer3 = ResidualBlock::new(vs, 128, 256);
let fc = nn::linear(vs, 256, 10, Default::default());
ResNet { conv1, bn1, layer1, layer2, layer3, fc }
}
}
impl nn::ModuleT for ResNet {
fn forward_t(&self, xs: &Tensor, train: bool) -> Tensor {
xs.apply(&self.conv1)
.apply_t(&self.bn1, train)
.relu()
.max_pool2d_default(2)
.apply_t(&self.layer1, train)
.apply_t(&self.layer2, train)
.apply_t(&self.layer3, train)
.adaptive_avg_pool2d(&[1, 1])
.view([-1, 256])
.apply(&self.fc)
}
}
// Define a Residual Block for ResNet.
#[derive(Debug)]
struct ResidualBlock {
conv1: nn::Conv2D,
bn1: nn::BatchNorm,
conv2: nn::Conv2D,
bn2: nn::BatchNorm,
shortcut: Option<nn::Conv2D>,
}
impl ResidualBlock {
fn new(vs: &nn::Path, in_channels: i64, out_channels: i64) -> ResidualBlock {
let conv1 = nn::conv2d(vs, in_channels, out_channels, 3, Default::default());
let bn1 = nn::batch_norm2d(vs, out_channels, Default::default());
let conv2 = nn::conv2d(vs, out_channels, out_channels, 3, Default::default());
let bn2 = nn::batch_norm2d(vs, out_channels, Default::default());
let shortcut = if in_channels != out_channels {
Some(nn::conv2d(vs, in_channels, out_channels, 1, Default::default()))
} else {
None
};
ResidualBlock { conv1, bn1, conv2, bn2, shortcut }
}
}
impl nn::ModuleT for ResidualBlock {
fn forward_t(&self, xs: &Tensor, train: bool) -> Tensor {
let shortcut = match &self.shortcut {
Some(sc) => xs.apply(sc),
None => xs.shallow_clone(),
};
xs.apply(&self.conv1)
.apply_t(&self.bn1, train)
.relu()
.apply(&self.conv2)
.apply_t(&self.bn2, train)
+ shortcut
}
}
// Function to train and test the ResNet model on the CIFAR-10 dataset.
fn run_resnet() -> Result<()> {
// Load the CIFAR-10 dataset.
let cifar_data = vision::cifar::load_dir("data/cifar10")?;
// Use GPU if available, otherwise use CPU.
let vs = nn::VarStore::new(Device::cuda_if_available());
let net = ResNet::new(&vs.root()); // Initialize the ResNet model.
let mut opt = nn::Adam::default().build(&vs, 1e-4)?; // Set up the optimizer.
// Reshape and normalize the training and test images.
let train_images = cifar_data.train_images / 255.0;
let train_labels = cifar_data.train_labels;
let test_images = cifar_data.test_images / 255.0;
let test_labels = cifar_data.test_labels;
// Training loop for the ResNet model.
for epoch in 1..=20 {
for (bimages, blabels) in train_images.split(128, 0).into_iter().zip(train_labels.split(128, 0).into_iter()) {
let loss = net.forward_t(&bimages, true).cross_entropy_for_logits(&blabels);
opt.backward_step(&loss); // Backpropagation step.
}
// Calculate and print test accuracy at the end of each epoch.
let test_accuracy = net.batch_accuracy_for_logits(&test_images, &test_labels, vs.device(), 256);
println!("Epoch: {:4}, Test Accuracy: {:5.2}%", epoch, 100. * test_accuracy);
}
Ok(())
}
The program begins by downloading and extracting the CIFAR-10 dataset if it is not already present. The ResNet
class is defined with three residual blocks and a fully connected layer for final classification, while each residual block contains convolutional layers with optional shortcuts to match dimensions. The main function initializes a single-threaded Tokio runtime to manage asynchronous tasks and calls the download_cifar10
function to ensure the dataset is available. The run_resnet
function loads the CIFAR-10 dataset, preprocesses the images by normalizing their pixel values, and sets up the ResNet model and optimizer. It then iterates over multiple epochs, training the model in batches by computing the loss and backpropagating the gradients. After each epoch, the model's accuracy on the test dataset is calculated and printed, providing insights into its performance improvement over time.
In industry, modern CNN architectures such as ResNet, Inception, and EfficientNet have become the backbone of various applications, ranging from image classification to object detection and medical image analysis. These architectures are often fine-tuned on domain-specific datasets using transfer learning, where pre-trained models (trained on large datasets like ImageNet) are adapted to new tasks by updating only the final layers. Rust’s ecosystem, with libraries like tch-rs
, supports loading and fine-tuning pre-trained models, allowing developers to leverage state-of-the-art CNN architectures in performance-sensitive environments, such as embedded systems, robotics, and autonomous vehicles.
In conclusion, modern CNN architectures represent the convergence of several innovations aimed at improving depth, efficiency, and performance. By building CNNs in Rust, developers can harness the power of these architectures in a highly efficient, safe, and performance-oriented programming environment, making Rust a compelling choice for deep learning in both research and industry applications.
6.2. Implementing VGG and Its Variants
The VGG architecture, introduced by Simonyan and Zisserman in 2014, is one of the most influential CNN architectures that emphasized the power of depth while maintaining simplicity. The fundamental design principle of VGG is straightforward: it uses small $3 \times 3$ filters consistently throughout the network while increasing the number of layers. This approach allows for the network to capture increasingly complex and abstract features with depth, without dramatically increasing the computational cost as seen with larger filters. The small filter size of $3 \times 3$ ensures that each convolutional layer has a limited receptive field, but by stacking many such layers, VGG can capture larger patterns and hierarchies over deeper layers.
Formally, a $3 \times 3$ convolutional filter can be represented as:
$$ y[i,j] = \sum_{m=0}^{2} \sum_{n=0}^{2} W[m,n] \cdot x[i+m,j+n] + b $$
where $x[i,j]$ is the input pixel value, $W[m,n]$ represents the filter weights, and $b$ is the bias term. This operation applies the filter over the input image, transforming it into a feature map. VGG extends this by applying multiple layers of such small filters, with each layer progressively learning more complex patterns.
The layer configuration of VGG follows a consistent pattern. It starts with several convolutional layers, followed by max pooling to reduce spatial dimensions, and ends with a series of fully connected layers for classification. VGG typically comes in variants such as VGG-16 and VGG-19, where the numbers refer to the total number of layers in the network. A typical VGG configuration for the convolutional layers would look like this: $3 \times 3$ convolutions with a stride of 1, followed by ReLU activation and max pooling.
One of the key insights behind VGG is that by stacking multiple small filters, the network can effectively replace the need for larger filters, which would otherwise have significantly more parameters and computational requirements. For example, two stacked $3 \times 3$ convolutions achieve a receptive field size equivalent to a $5 \times 5$ filter, while requiring fewer parameters. This stacking also adds more non-linearities, improving the model's ability to learn complex features.
While VGG's simplicity makes it easy to interpret and modify, it does have some trade-offs. The increase in depth, although beneficial for learning, makes the model computationally expensive in terms of memory and training time. VGG has a large number of parameters, particularly in its fully connected layers, which leads to higher memory usage. However, the simplicity of the architecture makes it easier to adapt and experiment with, which has led to many successful variants and improvements over the years.
From a conceptual perspective, the depth of VGG allows it to capture detailed and complex features from images. This depth makes VGG particularly effective on large datasets like ImageNet, where the abundance of features justifies the complexity of the network. However, there are performance trade-offs—while VGG performs exceptionally well in terms of accuracy, it is computationally heavy compared to later architectures such as ResNet and EfficientNet, which optimize for both depth and efficiency.
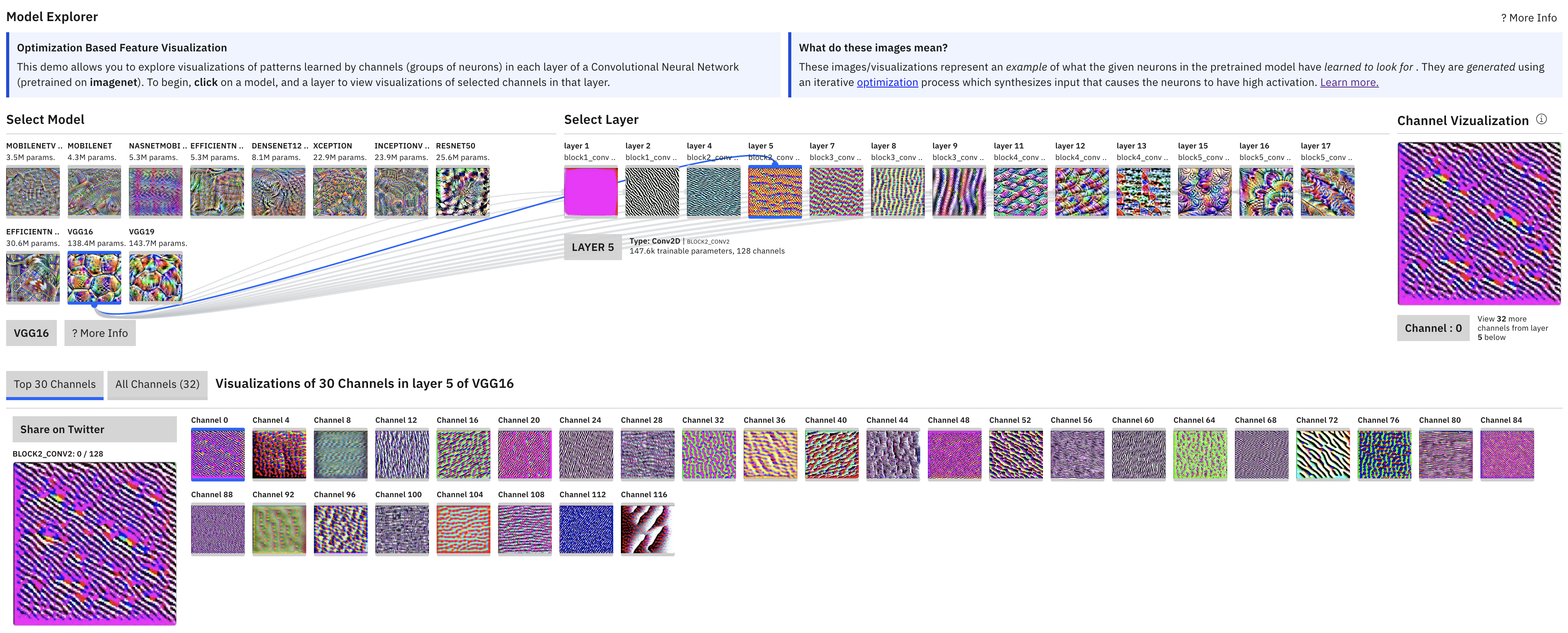
Figure 2: Model visualization of VGG16 architecture.
VGG-16 is a deep convolutional neural network architecture introduced by the Visual Geometry Group at the University of Oxford. It is renowned for its simplicity and uniform structure, consisting of sequential 3x3 convolutional layers with ReLU activations, followed by max-pooling layers and fully connected layers at the end. VGG-16 is widely used for image classification tasks, offering a balance between depth and computational efficiency.
The provided code implements the VGG-16 architecture to classify images in the CIFAR-10 dataset, a benchmark dataset consisting of 60,000 32x32 RGB images across 10 classes. It handles the download, extraction, and preprocessing of the CIFAR-10 dataset, followed by training and evaluating the VGG-16 model. The following code demonstrates how to implement a simplified version of VGG-16 in Rust:
use anyhow::{Result, Context};
use flate2::read::GzDecoder;
use reqwest;
use std::{fs, path::Path};
use tar::Archive;
use tch::{nn, nn::ModuleT, nn::OptimizerConfig, Device, Tensor, vision};
/// URL for the CIFAR-10 dataset
const CIFAR10_URL: &str = "https://www.cs.toronto.edu/~kriz/cifar-10-binary.tar.gz";
/// Function to download and extract the CIFAR-10 dataset.
async fn download_cifar10() -> Result<()> {
let dest = "data/cifar10";
if Path::new(&format!("{}/test_batch.bin", dest)).exists() {
println!("CIFAR-10 dataset already exists, skipping download.");
return Ok(());
}
fs::create_dir_all(dest).context("Failed to create data directory")?;
println!("Downloading CIFAR-10 dataset...");
let response = reqwest::get(CIFAR10_URL).await?;
let bytes = response.bytes().await?;
let tar_gz = GzDecoder::new(&bytes[..]);
let mut archive = Archive::new(tar_gz);
println!("Extracting CIFAR-10 dataset...");
for entry in archive.entries()? {
let mut entry = entry?;
let path = entry.path()?.into_owned();
// Extract only files into the correct directory
if let Some(file_name) = path.file_name() {
let file_path = Path::new(dest).join(file_name);
entry.unpack(file_path)?;
}
}
println!("CIFAR-10 dataset downloaded and extracted successfully.");
Ok(())
}
#[tokio::main]
async fn main() -> Result<()> {
// Ensure the CIFAR-10 dataset is downloaded and extracted.
download_cifar10().await?;
// Run the VGG-16 model training.
run_vgg16()
}
// Define the VGG-16 architecture.
#[derive(Debug)]
struct VGG16 {
features: nn::Sequential,
classifier: nn::Sequential,
}
impl VGG16 {
fn new(vs: &nn::Path) -> VGG16 {
let mut features = nn::seq();
let mut in_channels = 3;
// VGG-16 convolutional layers configuration.
let cfg: Vec<Option<i64>> = vec![
Some(64), Some(64), None, // MaxPooling
Some(128), Some(128), None,
Some(256), Some(256), Some(256), None,
Some(512), Some(512), Some(512), None,
Some(512), Some(512), Some(512), None,
];
for layer in cfg {
match layer {
Some(filters) => {
features = features
.add(nn::conv2d(
vs,
in_channels,
filters,
3,
nn::ConvConfig { padding: 1, ..Default::default() },
))
.add_fn(|x| x.relu());
in_channels = filters;
}
None => {
features = features.add_fn(|x| x.max_pool2d(&[2, 2], &[2, 2], &[0, 0], &[1, 1], false)); // MaxPooling
}
}
}
let classifier = nn::seq()
.add(nn::linear(vs, 512, 4096, Default::default()))
.add_fn(|x| x.relu())
.add_fn(|x| x.dropout(0.5, true))
.add(nn::linear(vs, 4096, 4096, Default::default()))
.add_fn(|x| x.relu())
.add_fn(|x| x.dropout(0.5, true))
.add(nn::linear(vs, 4096, 10, Default::default()));
VGG16 { features, classifier }
}
}
impl nn::ModuleT for VGG16 {
fn forward_t(&self, xs: &Tensor, train: bool) -> Tensor {
xs.apply(&self.features)
.view([-1, 512]) // Flatten
.apply_t(&self.classifier, train)
}
}
// Function to train and test the VGG-16 model on the CIFAR-10 dataset.
fn run_vgg16() -> Result<()> {
// Load the CIFAR-10 dataset.
let cifar_data = vision::cifar::load_dir("data/cifar10")?;
// Use GPU if available, otherwise use CPU.
let vs = nn::VarStore::new(Device::cuda_if_available());
let net = VGG16::new(&vs.root()); // Initialize the VGG-16 model.
let mut opt = nn::Adam::default().build(&vs, 1e-4)?; // Set up the optimizer.
// Reshape and normalize the training and test images.
let train_images = cifar_data.train_images / 255.0;
let train_labels = cifar_data.train_labels;
let test_images = cifar_data.test_images / 255.0;
let test_labels = cifar_data.test_labels;
// Training loop for the VGG-16 model.
for epoch in 1..=20 {
for (bimages, blabels) in train_images.split(128, 0).into_iter().zip(train_labels.split(128, 0).into_iter()) {
let loss = net.forward_t(&bimages, true).cross_entropy_for_logits(&blabels);
opt.backward_step(&loss); // Backpropagation step.
}
// Calculate and print test accuracy at the end of each epoch.
let test_accuracy = net.batch_accuracy_for_logits(&test_images, &test_labels, vs.device(), 256);
println!("Epoch: {:4}, Test Accuracy: {:5.2}%", epoch, 100. * test_accuracy);
}
Ok(())
}
The code begins by defining a function to download and extract the CIFAR-10 dataset, ensuring the required files are properly placed in the data/cifar10
directory. The VGG16
class implements the VGG-16 architecture, where convolutional layers are added sequentially based on a predefined configuration. Max-pooling layers are interspersed to reduce spatial dimensions, while fully connected layers handle the final classification. In the main
function, the dataset is downloaded and loaded using the tch
library. Images are normalized, and the model is trained for 20 epochs using the Adam optimizer. Training involves forward propagation, loss computation using cross-entropy, and backpropagation. After each epoch, the model's accuracy on the test set is evaluated and displayed, providing feedback on its performance.
The flexibility of the VGG architecture also allows for modifications. For instance, we can experiment with reducing the number of layers to create a shallower network, or we can adjust the filter sizes to see how the model’s performance changes. These modifications help in understanding the trade-offs between model complexity and performance.
From an industry perspective, VGG continues to be a benchmark architecture, particularly for tasks where interpretability and simplicity are prioritized. Its layer-wise design and regularity make it easy to modify, fine-tune, and experiment with, even though more recent architectures like ResNet and EfficientNet are preferred for computational efficiency. Despite its computational cost, VGG remains a popular choice in scenarios where accuracy is more critical than speed, especially when training is done on powerful hardware.
6.3. ResNet and the Power of Residual Connections
The ResNet (Residual Network) architecture represents a major leap forward in deep learning by addressing a critical issue in training deep neural networks: the vanishing gradient problem. This problem arises when the gradients (used to update weights during backpropagation) become extremely small as they propagate through the layers of a deep network, making it difficult to train very deep networks effectively. ResNet overcomes this limitation through the use of residual connections, or skip connections, which allow the network to bypass certain layers by directly feeding the input into later layers. This innovation enables the training of networks with hundreds of layers, such as ResNet-152, without suffering from the performance degradation typically associated with deeper networks.
Mathematically, the core idea behind ResNet can be formalized as follows: instead of learning an underlying mapping $H(x)$, the network learns a residual mapping $F(x) = H(x) - x$. This allows the model to reformulate the learning task as learning the difference (residual) between the input and the desired output:
$$ y = F(x) + x $$
Here, $F(x)$ represents the transformation learned by a series of convolutional layers, while $x$ is the original input that is added back to the output. This simple but powerful technique allows gradients to flow through the network more effectively, as the identity mapping xxx ensures that information is preserved across layers. In turn, this mitigates the vanishing gradient problem and enables the network to maintain accuracy even as the number of layers increases.
The skip connection mechanism has a profound impact on the stability and efficiency of training deep networks. By directly adding the input to the output of certain layers, ResNet allows gradients to propagate backward through the network more easily, ensuring that they do not diminish to near-zero values. This means that even very deep versions of ResNet, such as ResNet-152, can be trained without encountering the significant degradation in accuracy that would occur in traditional deep networks. This scalability is one of the defining features of ResNet, enabling architectures with depths ranging from ResNet-18 to ResNet-152 and beyond.
One of the key conceptual insights provided by ResNet is the understanding that residual connections allow networks to maintain the flow of information across layers. In traditional deep networks, the deeper the network, the harder it is for information from earlier layers to reach the output. Residual connections solve this by ensuring that at least some part of the input (the identity mapping) is directly passed through to the output. This is particularly important for very deep networks, where maintaining the integrity of information becomes increasingly difficult.
The identity mapping in ResNet plays a crucial role in this architecture. It ensures that the network can easily learn the identity function when needed, meaning that if a deeper layer does not need to transform the input, the network can simply pass the input through unchanged. This flexibility helps prevent overfitting, as the network can focus on learning useful transformations only when necessary.
ResNet's modularity also contributes to its scalability and adaptability. Each residual block is essentially a self-contained unit, consisting of a few convolutional layers and a skip connection. This modularity allows for easy extension of the network by simply adding more blocks, making it straightforward to build deeper models (such as ResNet-50 or ResNet-152) without needing to redesign the entire architecture. This modularity also allows ResNet to be adapted to a wide range of tasks, from image classification to object detection and segmentation.
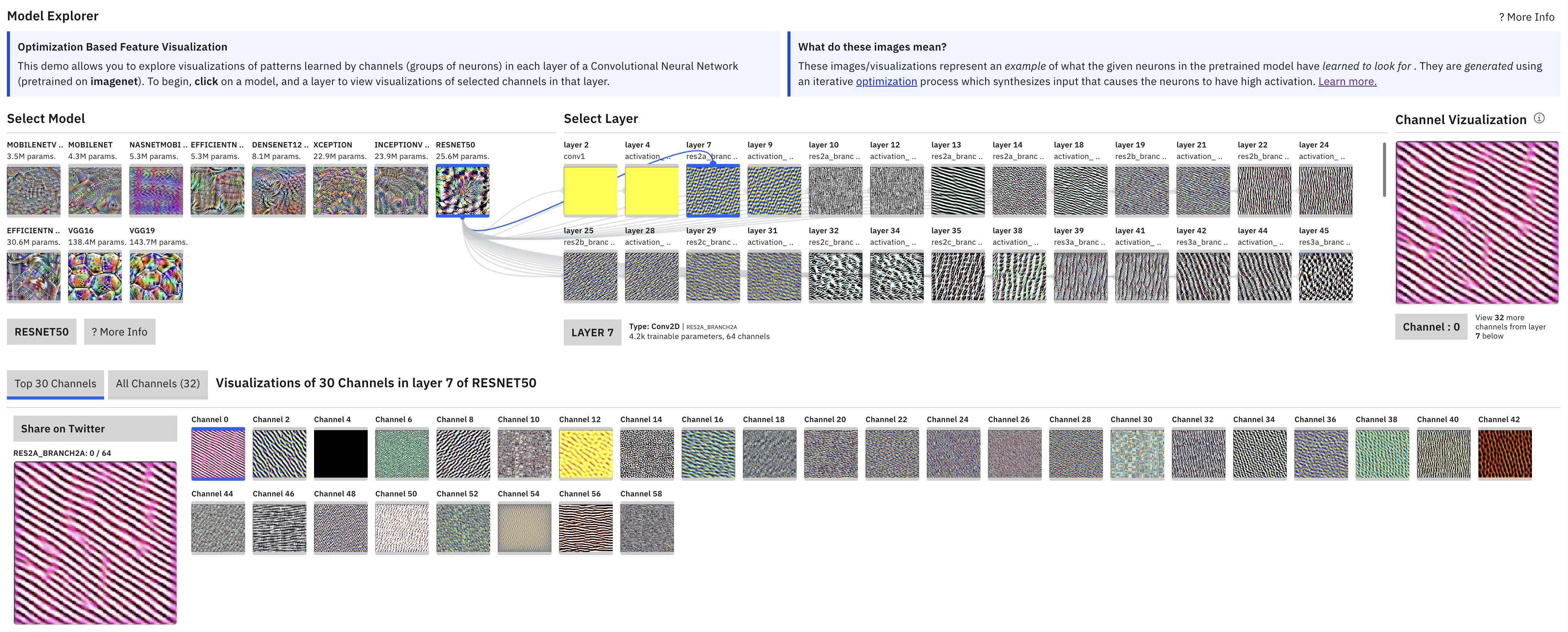
Figure 3: Model visualization of ResNet50 architecture.
Using Rust’s tch-rs
library, we can implement a simplified version of ResNet by defining residual blocks and stacking them to create deeper architectures. ResNet-50 is a deep architecture that introduces residual connections to help mitigate the vanishing gradient problem, allowing the network to be much deeper. It utilizes residual learning, where identity mappings (or shortcuts) skip layers, mitigating the vanishing gradient problem in deep networks. This enables deeper networks to be trained efficiently. ResNet-50 consists of 50 layers, including convolutional layers, batch normalization, ReLU activations, and fully connected layers, organized into a series of residual blocks. Each block uses skip connections to directly propagate input features, preserving information and improving gradient flow during training.
Below is the implementation of the ResNet-50 architecture using the tch-rs library for classifying images in the CIFAR-10 dataset. This implementation defines the ResNet-50 architecture, sets up the CIFAR-10 dataset, and uses the Adam optimizer for training. After training, we evaluate the model's accuracy on the test set.
use anyhow::{Result, Context};
use flate2::read::GzDecoder;
use reqwest;
use std::{fs, path::Path};
use tar::Archive;
use tch::{nn, nn::ModuleT, nn::OptimizerConfig, Device, Tensor, vision};
/// URL for the CIFAR-10 dataset
const CIFAR10_URL: &str = "https://www.cs.toronto.edu/~kriz/cifar-10-binary.tar.gz";
/// Function to download and extract the CIFAR-10 dataset.
async fn download_cifar10() -> Result<()> {
let dest = "data/cifar10";
if Path::new(&format!("{}/test_batch.bin", dest)).exists() {
println!("CIFAR-10 dataset already exists, skipping download.");
return Ok(());
}
fs::create_dir_all(dest).context("Failed to create data directory")?;
println!("Downloading CIFAR-10 dataset...");
let response = reqwest::get(CIFAR10_URL).await?;
let bytes = response.bytes().await?;
let tar_gz = GzDecoder::new(&bytes[..]);
let mut archive = Archive::new(tar_gz);
println!("Extracting CIFAR-10 dataset...");
archive.unpack("data")?;
println!("CIFAR-10 dataset downloaded and extracted successfully.");
Ok(())
}
#[tokio::main]
async fn main() -> Result<()> {
// Ensure the CIFAR-10 dataset is downloaded and extracted.
download_cifar10().await?;
// Run the ResNet-50 model training.
run_resnet50()
}
// Define the ResNet-50 architecture.
#[derive(Debug)]
struct ResNet50 {
conv1: nn::Conv2D,
bn1: nn::BatchNorm,
layers: nn::Sequential,
fc: nn::Linear,
}
impl ResNet50 {
fn new(vs: &nn::Path) -> ResNet50 {
let conv1 = nn::conv2d(
vs,
3,
64,
7,
nn::ConvConfig {
stride: 2,
padding: 3,
..Default::default()
},
);
let bn1 = nn::batch_norm2d(vs, 64, Default::default());
let mut layers = nn::seq();
layers = layers
.add(build_residual_block(vs, 64, 256, 3, 1))
.add(build_residual_block(vs, 256, 512, 4, 2))
.add(build_residual_block(vs, 512, 1024, 6, 2))
.add(build_residual_block(vs, 1024, 2048, 3, 2));
let fc = nn::linear(vs, 2048, 10, Default::default());
ResNet50 {
conv1,
bn1,
layers,
fc,
}
}
}
impl nn::ModuleT for ResNet50 {
fn forward_t(&self, xs: &Tensor, train: bool) -> Tensor {
xs.apply(&self.conv1)
.apply_t(&self.bn1, train)
.relu()
.max_pool2d(&[3, 3], &[2, 2], &[1, 1], &[1, 1], false)
.apply_t(&self.layers, train)
.adaptive_avg_pool2d(&[1, 1])
.view([-1, 2048])
.apply(&self.fc)
}
}
// Define the Residual Block.
#[derive(Debug)]
struct ResidualBlock {
conv1: nn::Conv2D,
bn1: nn::BatchNorm,
conv2: nn::Conv2D,
bn2: nn::BatchNorm,
conv3: nn::Conv2D,
bn3: nn::BatchNorm,
shortcut: Option<nn::Conv2D>,
}
impl ResidualBlock {
fn new(
vs: &nn::Path,
in_channels: i64,
out_channels: i64,
stride: i64,
shortcut: Option<nn::Conv2D>,
) -> ResidualBlock {
let conv1 = nn::conv2d(vs, in_channels, out_channels / 4, 1, Default::default());
let bn1 = nn::batch_norm2d(vs, out_channels / 4, Default::default());
let conv2 = nn::conv2d(
vs,
out_channels / 4,
out_channels / 4,
3,
nn::ConvConfig { stride, padding: 1, ..Default::default() },
);
let bn2 = nn::batch_norm2d(vs, out_channels / 4, Default::default());
let conv3 = nn::conv2d(vs, out_channels / 4, out_channels, 1, Default::default());
let bn3 = nn::batch_norm2d(vs, out_channels, Default::default());
ResidualBlock {
conv1,
bn1,
conv2,
bn2,
conv3,
bn3,
shortcut,
}
}
fn forward(&self, xs: &Tensor, train: bool) -> Tensor {
let shortcut = match &self.shortcut {
Some(sc) => xs.apply(sc),
None => xs.shallow_clone(),
};
xs.apply(&self.conv1)
.apply_t(&self.bn1, train)
.relu()
.apply(&self.conv2)
.apply_t(&self.bn2, train)
.relu()
.apply(&self.conv3)
.apply_t(&self.bn3, train)
+ shortcut
}
}
// Wrapper for ResidualBlock
#[derive(Debug)]
struct ResidualBlockWrapper {
block: ResidualBlock,
}
impl ResidualBlockWrapper {
fn new(
vs: &nn::Path,
in_channels: i64,
out_channels: i64,
stride: i64,
shortcut: Option<nn::Conv2D>,
) -> Self {
Self {
block: ResidualBlock::new(vs, in_channels, out_channels, stride, shortcut),
}
}
}
impl nn::Module for ResidualBlockWrapper {
fn forward(&self, xs: &Tensor) -> Tensor {
self.block.forward(xs, false)
}
}
// Function to build a series of residual blocks.
fn build_residual_block(
vs: &nn::Path,
in_channels: i64,
out_channels: i64,
blocks: i64,
stride: i64,
) -> nn::Sequential {
let mut layer = nn::seq();
let shortcut = if stride != 1 || in_channels != out_channels {
Some(nn::conv2d(
vs,
in_channels,
out_channels,
1,
nn::ConvConfig {
stride,
..Default::default()
},
))
} else {
None
};
layer = layer.add(ResidualBlockWrapper::new(
vs,
in_channels,
out_channels,
stride,
shortcut,
));
for _ in 1..blocks {
layer = layer.add(ResidualBlockWrapper::new(vs, out_channels, out_channels, 1, None));
}
layer
}
// Function to train and test the ResNet-50 model on the CIFAR-10 dataset.
fn run_resnet50() -> Result<()> {
// Load the CIFAR-10 dataset.
let cifar_data = vision::cifar::load_dir("data/cifar10")?;
// Use GPU if available, otherwise use CPU.
let vs = nn::VarStore::new(Device::cuda_if_available());
let net = ResNet50::new(&vs.root()); // Initialize the ResNet-50 model.
let mut opt = nn::Sgd::default().build(&vs, 0.01)?; // Set up SGD optimizer.
// Reshape and normalize the training and test images.
let train_images = cifar_data.train_images / 255.0;
let train_labels = cifar_data.train_labels;
let test_images = cifar_data.test_images / 255.0;
let test_labels = cifar_data.test_labels;
// Training loop for the ResNet-50 model.
for epoch in 1..=20 {
for (bimages, blabels) in train_images.split(128, 0).into_iter().zip(train_labels.split(128, 0).into_iter()) {
let loss = net.forward_t(&bimages, true).cross_entropy_for_logits(&blabels);
opt.backward_step(&loss); // Backpropagation step.
}
// Calculate and print test accuracy at the end of each epoch.
let test_accuracy = net.batch_accuracy_for_logits(&test_images, &test_labels, vs.device(), 256);
println!("Epoch: {:4}, Test Accuracy: {:5.2}%", epoch, 100. * test_accuracy);
}
Ok(())
}
The code implements a ResNet-50 model for classifying images in the CIFAR-10 dataset. It begins by downloading and preparing the CIFAR-10 dataset. The ResNet50
class defines the architecture, including initial convolutional and batch normalization layers, multiple residual block layers, and a fully connected output layer for classification. Residual blocks are encapsulated in the ResidualBlock
and ResidualBlockWrapper
structs, enabling compatibility with the nn::Sequential
API. The training loop loads batches of CIFAR-10 images, computes loss using cross-entropy, and updates model weights using stochastic gradient descent (SGD). Test accuracy is evaluated after each epoch, demonstrating the model's performance. The implementation leverages the tch
library for PyTorch-like tensor operations and neural network building in Rust.
ResNet is a family of CNN architectures designed to mitigate the vanishing gradient problem through residual connections. The different variants, such as ResNet18, ResNet34, ResNet50, ResNet101, and ResNet152, differ primarily in the depth of the network, i.e., the number of layers. ResNet18 has 18 layers, ResNet34 has 34 layers, ResNet50 features 50 layers, ResNet101 has 101 layers, and ResNet152 is the deepest with 152 layers. These variants offer a trade-off between computational cost and accuracy, with deeper models generally achieving higher performance at the expense of increased complexity and training time.
By experimenting with different ResNet depths (e.g., ResNet-18, ResNet-50, ResNet-101), we can analyze the trade-offs between depth, accuracy, and computational cost. Deeper networks tend to perform better on larger, more complex datasets, but they also require more computational resources in terms of memory and processing power. Shallower networks, like ResNet-18, may be sufficient for smaller datasets or less complex tasks, offering a good balance between performance and efficiency.
[dependencies]
accelerate-src = "0.3.2"
anyhow = "1.0"
candle-core = "0.8.0"
candle-examples = "0.8.0"
candle-nn = "0.8.0"
candle-transformers = "0.8.0"
clap = { version = "4", features = ["derive"] }
hf-hub = "0.3.2"
use candle_core::{DType, IndexOp, D};
use candle_nn::{Module, VarBuilder};
use candle_transformers::models::resnet;
use clap::{Parser, ValueEnum};
#[derive(Clone, Copy, Debug, ValueEnum)]
enum Which {
#[value(name = "18")]
Resnet18,
#[value(name = "34")]
Resnet34,
#[value(name = "50")]
Resnet50,
#[value(name = "101")]
Resnet101,
#[value(name = "152")]
Resnet152,
}
#[derive(Parser)]
struct Args {
#[clap(long)]
model: Option<String>,
#[clap(long)]
image: String,
/// Run on CPU rather than on GPU.
#[clap(long)]
cpu: bool,
/// Variant of the model to use.
#[clap(value_enum, long, default_value_t = Which::Resnet18)]
which: Which,
}
pub fn main() -> anyhow::Result<()> {
let args = Args::parse();
let device = candle_examples::device(args.cpu)?;
let image = candle_examples::imagenet::load_image224(args.image)?.to_device(&device)?;
println!("loaded image {image:?}");
let model_file = match args.model {
None => {
let api = hf_hub::api::sync::Api::new()?;
let api = api.model("lmz/candle-resnet".into());
let filename = match args.which {
Which::Resnet18 => "resnet18.safetensors",
Which::Resnet34 => "resnet34.safetensors",
Which::Resnet50 => "resnet50.safetensors",
Which::Resnet101 => "resnet101.safetensors",
Which::Resnet152 => "resnet152.safetensors",
};
api.get(filename)?
}
Some(model) => model.into(),
};
let vb = unsafe { VarBuilder::from_mmaped_safetensors(&[model_file], DType::F32, &device)? };
let class_count = candle_examples::imagenet::CLASS_COUNT as usize;
let model = match args.which {
Which::Resnet18 => resnet::resnet18(class_count, vb)?,
Which::Resnet34 => resnet::resnet34(class_count, vb)?,
Which::Resnet50 => resnet::resnet50(class_count, vb)?,
Which::Resnet101 => resnet::resnet101(class_count, vb)?,
Which::Resnet152 => resnet::resnet152(class_count, vb)?,
};
println!("model built");
let logits = model.forward(&image.unsqueeze(0)?)?;
let prs = candle_nn::ops::softmax(&logits, D::Minus1)?
.i(0)?
.to_vec1::<f32>()?;
let mut prs = prs.iter().enumerate().collect::<Vec<_>>();
prs.sort_by(|(_, p1), (_, p2)| p2.total_cmp(p1));
for &(category_idx, pr) in prs.iter().take(5) {
println!(
"{:24}: {:.2}%",
candle_examples::imagenet::CLASSES[category_idx],
100. * pr
);
}
Ok(())
}
In the sample codes, the candle
crate is used for defining and training these models using Rust. candle_nn
provides the neural network modules and operations, while candle_transformers
includes the pre-defined ResNet models. The code uses the clap
crate for argument parsing, allowing users to specify the image file, the model variant (ResNet18, ResNet34, etc.), and whether to run on a CPU or GPU. It loads the image, fetches the model weights, and uses the VarBuilder
to load the model into memory. The selected ResNet variant is then initialized and used to classify the image. The classification output (logits) is passed through a softmax operation to obtain probabilities, and the top 5 predicted categories are displayed with their respective probabilities. This implementation showcases a practical use of Rust for running pre-trained deep learning models.
From an industry perspective, ResNet has become a standard architecture for image recognition tasks and is widely used in various applications, from medical imaging to autonomous vehicles. Its modular design, scalability, and ability to train deep networks without performance degradation have made it one of the most successful and widely adopted architectures in deep learning.
In conclusion, ResNet’s introduction of residual connections has revolutionized the training of deep networks, making it possible to build models that are both deep and highly performant. By implementing and experimenting with ResNet in Rust, developers can take advantage of this architecture's powerful design principles while benefiting from Rust’s performance and memory safety features, making it ideal for applications that require both speed and reliability.
6.4. Inception Networks and Multi-Scale Feature Extraction
Inception networks represent a significant advancement in Convolutional Neural Network (CNN) architectures by introducing the concept of multi-scale feature extraction within a single layer. The core idea of Inception networks is to process input data at multiple scales simultaneously by applying various convolutional and pooling operations in parallel. This approach enables the model to capture both fine-grained details and broader, coarser patterns within the same layer, which is especially important when the features in the data vary widely in size. The flexibility and efficiency of Inception networks have made them highly effective for tasks like image classification and object detection.
The original Inception module (also known as Inception v1) is composed of multiple parallel paths, each of which applies a different type of operation to the input data. These paths include $1 \times 1$, $3 \times 3$, and $5 \times 5$ convolutions, along with a max pooling operation. The results from these different paths are concatenated together to form the output. Mathematically, if $x$ represents the input and $f_{1 \times 1}(x), f_{3 \times 3}(x), f_{5 \times 5}(x)$, and pooling $p(x)$ are the outputs from the different paths, the output of the Inception module is the concatenation of these results:
$$ y = \text{Concat}(f_{1 \times 1}(x), f_{3 \times 3}(x), f_{5 \times 5}(x), p(x)) $$
This approach allows the network to learn both local features (using smaller filters) and global patterns (using larger filters) simultaneously, providing a robust mechanism for handling a wide variety of feature sizes in the data.
A key innovation in the Inception v1 architecture was the use of $1 \times 1$ convolutions to reduce the dimensionality of the input before applying larger filters like $3 \times 3$ and $5 \times 5$. This dimensionality reduction technique significantly improves the computational efficiency of the network by reducing the number of input channels for the larger convolutions, thereby lowering the number of parameters and the computational cost. Formally, a $1 \times 1$ convolution can be expressed as:
$$ y[i,j] = \sum_{c=1}^{C} W_c x[i,j,c] $$
where $x[i,j,c]$ represents the input pixel value at location $(i,j)$ and channel $c$, and $W_c$ is the learned weight for channel $c$. This operation reduces the number of channels in the input while preserving the spatial resolution, making it an effective tool for controlling the computational complexity of the network.
The evolution of Inception modules from v1 to v4 introduced various refinements and optimizations to further improve both performance and efficiency. For example, Inception v2 introduced factorized convolutions, where larger filters (like $5 \times 5$) are replaced with multiple smaller filters (such as two $3 \times 3$ convolutions). This reduces the number of parameters while maintaining the network's ability to capture larger-scale patterns. Inception v3 and v4 continued this trend, introducing additional architectural improvements, such as using batch normalization to stabilize training and label smoothing to prevent overfitting. The Inception-ResNet variant combined the strengths of Inception with the residual connections from ResNet, allowing for even deeper and more powerful networks.
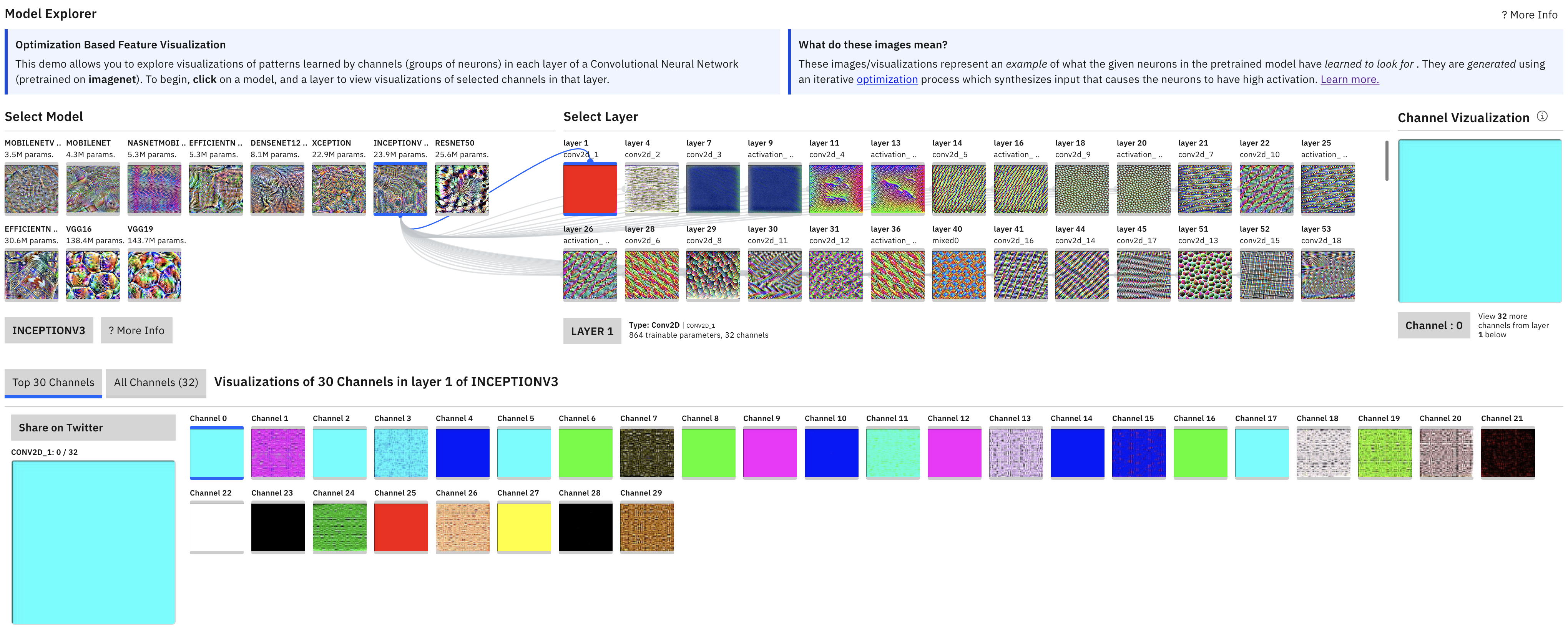
Figure 4: Visualization of InceptionV3 architecture.
From a conceptual perspective, the multi-scale feature extraction provided by Inception modules is critical for handling complex patterns in data. By applying convolutions with different filter sizes in parallel, the network can learn to recognize features of varying sizes without needing to manually tune the filter sizes for specific tasks. This architectural diversity within the same layer allows Inception networks to capture both fine details and broader structures, making them highly effective for tasks that require a nuanced understanding of the input data, such as object detection or scene recognition.
At the same time, Inception networks strike a balance between computational efficiency and model performance. The use of dimensionality reduction with $1 \times 1$ convolutions significantly reduces the number of parameters, making the network more efficient without sacrificing performance. This makes Inception networks particularly well-suited for tasks that require high accuracy but also need to be computationally feasible, such as deployment in resource-constrained environments like mobile devices.
The InceptionV4 architecture builds upon the strengths of previous Inception networks by integrating residual connections to improve gradient flow, making it highly scalable for deep networks. It consists of multiple modules: Stem, Inception-A, Inception-B, Inception-C, Reduction-A, and Reduction-B. The Stem acts as a feature extractor, while the Inception modules focus on efficiently capturing spatial features by combining convolutions of different sizes. The Reduction modules downsample the spatial dimensions and increase feature richness. This modular design enables effective representation learning, making InceptionV4 a powerful model for image classification tasks.
use anyhow::{Result, Context};
use flate2::read::GzDecoder;
use reqwest;
use std::{fs, path::Path};
use tar::Archive;
use tch::{nn, nn::ModuleT, nn::OptimizerConfig, Device, Tensor, vision};
/// URL for the CIFAR-10 dataset
const CIFAR10_URL: &str = "https://www.cs.toronto.edu/~kriz/cifar-10-binary.tar.gz";
/// Function to download and extract the CIFAR-10 dataset.
async fn download_cifar10() -> Result<()> {
let dest = "data/cifar10";
if Path::new(dest).exists() {
println!("CIFAR-10 dataset already exists, skipping download.");
return Ok(());
}
fs::create_dir_all(dest).context("Failed to create data directory")?;
println!("Downloading CIFAR-10 dataset...");
let response = reqwest::get(CIFAR10_URL).await?;
let bytes = response.bytes().await?;
let tar_gz = GzDecoder::new(&bytes[..]);
let mut archive = Archive::new(tar_gz);
println!("Extracting CIFAR-10 dataset...");
archive.unpack("data")?;
println!("CIFAR-10 dataset downloaded and extracted successfully.");
Ok(())
}
/// Define the Inception V4 architecture.
#[derive(Debug)]
struct InceptionV4 {
stem: nn::Sequential,
inception_a: nn::Sequential,
inception_b: nn::Sequential,
inception_c: nn::Sequential,
reduction_a: nn::Sequential,
reduction_b: nn::Sequential,
fc: nn::Linear,
}
impl InceptionV4 {
fn new(vs: &nn::Path) -> InceptionV4 {
let stem = nn::seq()
.add(nn::conv2d(vs, 3, 32, 3, Default::default()))
.add(nn::conv2d(vs, 32, 32, 3, nn::ConvConfig { stride: 2, ..Default::default() }))
.add(nn::conv2d(vs, 32, 64, 3, nn::ConvConfig { padding: 1, ..Default::default() }));
let inception_a = InceptionV4::inception_a_block(vs, 64, 32);
let inception_b = InceptionV4::inception_b_block(vs, 192, 64);
let inception_c = InceptionV4::inception_c_block(vs, 384, 128);
let reduction_a = InceptionV4::reduction_a_block(vs, 192);
let reduction_b = InceptionV4::reduction_b_block(vs, 384);
let fc = nn::linear(vs, 1024, 10, Default::default());
InceptionV4 {
stem,
inception_a,
inception_b,
inception_c,
reduction_a,
reduction_b,
fc,
}
}
fn inception_a_block(vs: &nn::Path, in_channels: i64, pool_channels: i64) -> nn::Sequential {
nn::seq()
.add(nn::conv2d(vs, in_channels, 64, 1, Default::default()))
.add(nn::conv2d(vs, in_channels, 48, 1, Default::default()))
.add(nn::conv2d(vs, 48, 64, 5, nn::ConvConfig { padding: 2, ..Default::default() }))
.add(nn::conv2d(vs, in_channels, 64, 1, Default::default()))
.add(nn::conv2d(vs, 64, 96, 3, nn::ConvConfig { padding: 1, ..Default::default() }))
.add(nn::conv2d(vs, 96, 96, 3, nn::ConvConfig { padding: 1, ..Default::default() }))
.add(nn::conv2d(vs, in_channels, pool_channels, 1, Default::default()))
}
fn inception_b_block(vs: &nn::Path, in_channels: i64, channels: i64) -> nn::Sequential {
nn::seq()
.add(nn::conv2d(vs, in_channels, channels, 1, Default::default()))
.add(nn::conv2d(vs, channels, channels, 7, nn::ConvConfig { padding: 3, ..Default::default() }))
}
fn inception_c_block(vs: &nn::Path, in_channels: i64, channels: i64) -> nn::Sequential {
nn::seq()
.add(nn::conv2d(vs, in_channels, channels, 1, Default::default()))
.add(nn::conv2d(vs, channels, channels, 3, nn::ConvConfig { padding: 1, ..Default::default() }))
}
fn reduction_a_block(vs: &nn::Path, in_channels: i64) -> nn::Sequential {
nn::seq()
.add(nn::conv2d(vs, in_channels, 384, 3, nn::ConvConfig { stride: 2, ..Default::default() }))
.add(nn::conv2d(vs, in_channels, 64, 1, Default::default()))
.add(nn::conv2d(vs, 64, 96, 3, nn::ConvConfig { padding: 1, ..Default::default() }))
.add(nn::conv2d(vs, 96, 96, 3, nn::ConvConfig { stride: 2, ..Default::default() }))
}
fn reduction_b_block(vs: &nn::Path, in_channels: i64) -> nn::Sequential {
nn::seq()
.add(nn::conv2d(vs, in_channels, 192, 1, Default::default()))
.add(nn::conv2d(vs, 192, 320, 3, nn::ConvConfig { stride: 2, ..Default::default() }))
}
}
impl nn::ModuleT for InceptionV4 {
fn forward_t(&self, xs: &Tensor, train: bool) -> Tensor {
xs.apply_t(&self.stem, train)
.apply_t(&self.inception_a, train)
.apply_t(&self.reduction_a, train)
.apply_t(&self.inception_b, train)
.apply_t(&self.reduction_b, train)
.apply_t(&self.inception_c, train)
.adaptive_avg_pool2d(&[1, 1])
.view([-1, 1024])
.apply(&self.fc)
}
}
/// Train and test the Inception V4 model on CIFAR-10.
fn run_inception_v4() -> Result<()> {
// Load CIFAR-10 dataset
let cifar_data = vision::cifar::load_dir("data/cifar10")?;
// Use GPU if available, otherwise CPU
let vs = nn::VarStore::new(Device::cuda_if_available());
let net = InceptionV4::new(&vs.root());
let mut opt = nn::Adam::default().build(&vs, 1e-3)?;
let train_images = cifar_data.train_images / 255.0;
let train_labels = cifar_data.train_labels;
let test_images = cifar_data.test_images / 255.0;
let test_labels = cifar_data.test_labels;
// Training loop
for epoch in 1..=20 {
for (bimages, blabels) in train_images.split(128, 0).into_iter().zip(train_labels.split(128, 0).into_iter()) {
let loss = net.forward_t(&bimages, true).cross_entropy_for_logits(&blabels);
opt.backward_step(&loss);
}
// Test accuracy
let test_accuracy = net.batch_accuracy_for_logits(&test_images, &test_labels, vs.device(), 256);
println!("Epoch: {:4}, Test Accuracy: {:5.2}%", epoch, 100. * test_accuracy);
}
Ok(())
}
#[tokio::main]
async fn main() -> Result<()> {
download_cifar10().await?;
run_inception_v4()
}
The provided code is a Rust program that downloads the CIFAR-10 dataset, extracts it, and trains an Inception V4 model on the dataset using the tch
crate, which provides bindings for PyTorch in Rust. The download_cifar10
function asynchronously fetches the CIFAR-10 dataset from the specified URL, extracts the tar.gz file using flate2
and tar
crates, and saves the dataset to the local "data/cifar10" directory. The InceptionV4
struct defines the architecture of the Inception V4 model, with various layers including convolutions, inception blocks, and reductions. The forward_t
method implements the forward pass through the network. The run_inception_v4
function sets up the model, optimizer, and training loop, and then trains the model for 20 epochs using the CIFAR-10 training set while evaluating the model's accuracy on the test set after each epoch. The main
function orchestrates the process by first downloading the dataset and then calling the training function. This implementation utilizes GPU if available, thanks to the Device::cuda_if_available
function from the tch
crate, enabling accelerated computation for deep learning tasks.
To fully leverage the power of Inception networks, training them on large, complex datasets like ImageNet is essential, as their multi-scale feature extraction capabilities allow them to capture both detailed and abstract features from diverse image sets. Inception networks are particularly advantageous due to their ability to achieve high accuracy while remaining computationally efficient, thanks to techniques like dimensionality reduction and factorized convolutions. This balance between performance and efficiency makes them ideal for large-scale applications in industry, such as image classification, object detection, and real-time video analysis, where both accuracy and speed are critical. Additionally, developers can experiment with custom Inception modules by modifying paths and operations, such as replacing 5x5 convolutions with consecutive 3x3 convolutions to reduce parameters or adding dilated convolutions to capture larger-scale features. By exploring these variations, developers can optimize Inception networks for specific tasks or datasets, enhancing their performance, efficiency, or both.
In summary, Inception networks have become a cornerstone of modern CNN architectures, providing a powerful mechanism for extracting features at multiple scales within a single layer. Through innovations such as multi-scale feature extraction and dimensionality reduction, Inception networks strike a balance between computational efficiency and model performance, making them highly versatile for a wide range of applications. Implementing Inception modules in Rust allows developers to explore these concepts while benefiting from Rust’s efficiency and safety features, making it an excellent choice for high-performance machine learning applications.
6.5. DenseNet and Feature Reuse
The DenseNet (Densely Connected Convolutional Networks) architecture is a modern CNN design that introduces the concept of dense connectivity between layers. Unlike traditional CNNs, where each layer passes its output to the next layer sequentially, DenseNet connects each layer to every other layer in a feed-forward fashion. This design encourages feature reuse, allowing the network to propagate both the original input and the learned features from previous layers forward through the network. As a result, DenseNet achieves high accuracy with fewer parameters and improved gradient flow, making it highly efficient.
Mathematically, the output of the $l^{th}$ layer in a DenseNet is defined as:
$$x_l = H_l([x_0, x_1, ..., x_{l-1}])$$
where $[x_0, x_1, ..., x_{l-1}]$ represents the concatenation of the feature maps from all previous layers, and $H_l(\cdot)$ is the operation (e.g., convolution, activation) applied at the current layer. This formulation contrasts with traditional CNNs, where each layer receives only the output of the previous layer. By concatenating the outputs of all preceding layers, DenseNet promotes feature reuse, allowing the model to use earlier features in later stages of the network.
One of the key components of DenseNet is the concept of a growth rate. The growth rate controls how much information each new layer contributes to the network. A smaller growth rate means that each layer adds a limited number of new feature maps, leading to more compact models. Conversely, a larger growth rate increases the number of features learned by each layer, potentially improving performance but at the cost of increased model size. The number of feature maps at layer lll in a DenseNet is given by:
$$f_l = f_0 + l \cdot k$$
where $f_0$ is the number of input feature maps, $l$ is the layer index, and $k$ is the growth rate. This linear growth ensures that the model remains manageable in size, even as the depth increases.
The dense blocks in DenseNet, which consist of several layers with dense connectivity, play a crucial role in improving gradient flow and feature propagation. By ensuring that each layer receives the gradients from all preceding layers, DenseNet mitigates the vanishing gradient problem and allows for more effective training of deep networks. Additionally, by reusing features across layers, DenseNet reduces the need to learn redundant features, leading to more parameter-efficient models.
From a conceptual perspective, DenseNet’s dense connectivity promotes feature reuse, which is a key innovation that reduces the total number of parameters while maintaining high accuracy. In traditional networks, each layer learns new features independently, often resulting in redundant feature extraction. DenseNet, by contrast, allows each layer to build on previously learned features, ensuring that the model can extract and propagate important features throughout the network. This reuse of features also enables DenseNet to achieve competitive performance with fewer parameters compared to architectures like ResNet.
The growth rate is an important hyperparameter in DenseNet that balances model complexity and performance. By controlling how many new features each layer adds, the growth rate influences the size of the model and its ability to learn. A smaller growth rate results in more compact models with fewer parameters, while a larger growth rate increases the representational capacity of the model but also its computational requirements. In practice, choosing the right growth rate depends on the specific task and the computational resources available.
Another advantage of DenseNet is its ability to achieve high accuracy with fewer parameters. By reusing features across layers, DenseNet reduces the redundancy that often arises in deeper networks, allowing the model to learn more efficiently. This is particularly valuable in applications where computational resources are limited, as DenseNet can deliver strong performance without requiring excessively large models.
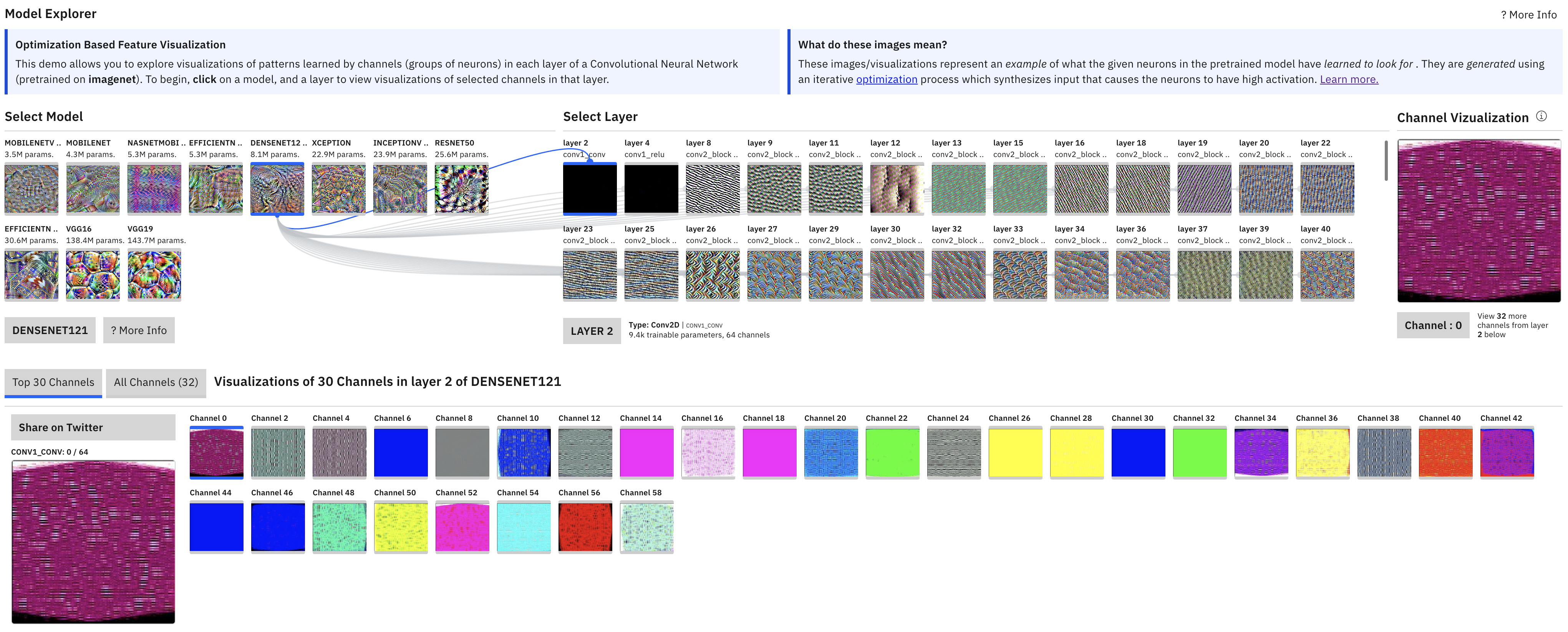
Figure 5: Visualization of DenseNet121 architecture.
Here’s the implementation of the DenseNet-121 architecture using the tch-rs library for the ImageNet dataset. DenseNet is a deep learning architecture where each layer is connected to every other layer in a feed-forward manner. This connectivity encourages feature reuse, making the network more efficient and reducing the number of parameters. DenseNet-121 leverages dense connectivity between layers. Unlike traditional CNNs, where each layer receives input only from the previous layer, DenseNet uses a dense block where each layer receives input from all previous layers in that block. This results in more efficient use of features and a reduction in the number of parameters. DenseNet-121 specifically refers to a version of the DenseNet architecture with 121 layers. It includes multiple dense blocks, transition layers to reduce feature map dimensions, and a final global average pooling layer followed by a classifier for tasks like image classification.
use tch::{nn, nn::ModuleT, Device, Tensor};
/// Define the DenseNet-121 architecture.
#[derive(Debug)]
struct DenseNet121 {
features: nn::Sequential,
classifier: nn::Linear,
}
impl DenseNet121 {
fn new(vs: &nn::Path) -> DenseNet121 {
let mut features = nn::seq();
// Initial Convolution
features = features
.add(nn::conv2d(vs, 3, 64, 7, nn::ConvConfig { stride: 2, padding: 3, ..Default::default() }))
.add(nn::batch_norm2d(vs, 64, Default::default()))
.add_fn(|x| x.relu())
.add_fn(|x| x.max_pool2d(&[3, 3], &[2, 2], &[1, 1], &[1, 1], false));
// Dense Blocks and Transition Layers
features = features
.add(Self::dense_block(vs, 6, 64, 32))
.add(Self::transition_layer(vs, 256, 128))
.add(Self::dense_block(vs, 12, 128, 32))
.add(Self::transition_layer(vs, 512, 256))
.add(Self::dense_block(vs, 24, 256, 32))
.add(Self::transition_layer(vs, 1024, 512))
.add(Self::dense_block(vs, 16, 512, 32))
.add_fn(|x| x.adaptive_avg_pool2d(&[1, 1]));
// Classifier
let classifier = nn::linear(vs, 512, 10, Default::default());
DenseNet121 { features, classifier }
}
fn dense_block(vs: &nn::Path, num_layers: usize, in_channels: i64, growth_rate: i64) -> nn::Sequential {
let mut block = nn::seq();
let mut channels = in_channels;
for _ in 0..num_layers {
block = block.add(Self::dense_layer(vs, channels, growth_rate));
channels += growth_rate;
}
block
}
fn dense_layer(vs: &nn::Path, in_channels: i64, growth_rate: i64) -> nn::Sequential {
nn::seq()
.add(nn::batch_norm2d(vs, in_channels, Default::default()))
.add_fn(|x| x.relu())
.add(nn::conv2d(vs, in_channels, growth_rate, 3, nn::ConvConfig { padding: 1, ..Default::default() }))
}
fn transition_layer(vs: &nn::Path, in_channels: i64, out_channels: i64) -> nn::Sequential {
nn::seq()
.add(nn::batch_norm2d(vs, in_channels, Default::default()))
.add_fn(|x| x.relu())
.add(nn::conv2d(vs, in_channels, out_channels, 1, Default::default()))
.add_fn(|x| x.avg_pool2d(&[2, 2], &[2, 2], &[0, 0], &[1, 1], false))
}
}
impl nn::ModuleT for DenseNet121 {
fn forward_t(&self, xs: &Tensor, train: bool) -> Tensor {
xs.apply_t(&self.features, train)
.view([-1, 512])
.apply(&self.classifier)
}
}
The code snippet defines the DenseNet-121 architecture using the Rust library tch
, which provides tools for deep learning. It starts by defining a structure for the network, DenseNet121
, which includes two main components: features
(a sequential block of layers) and classifier
(a fully connected layer). The features
block includes an initial convolution layer, followed by dense blocks, transition layers, and global average pooling. The dense_block
function builds a block of layers where each new layer is connected to all previous ones, while the transition_layer
function reduces the spatial dimensions of the feature maps. Finally, the forward_t
method implements the forward pass, applying the feature layers followed by the classifier to produce the output.
When experimenting with DenseNet, developers can adjust the growth rate and the number of layers in each dense block to optimize the model for different tasks. A smaller growth rate will result in a more compact model with fewer parameters, while a larger growth rate will increase the model’s representational power. However, care must be taken to balance model complexity with computational efficiency, especially when deploying models in resource-constrained environments.
DenseNet is widely used in industry due to its ability to achieve high accuracy with relatively few parameters, making it ideal for applications where both performance and efficiency are critical. For example, DenseNet has been applied in medical imaging tasks, where accurate feature extraction is crucial, but computational resources may be limited. DenseNet’s compactness and efficiency also make it a popular choice for real-time applications, such as video analysis and autonomous systems.
In conclusion, DenseNet’s dense connectivity and feature reuse enable it to achieve high accuracy with fewer parameters, making it a highly efficient architecture for deep learning. Through the use of growth rates, DenseNet balances model size and performance, and its dense blocks promote effective gradient flow and feature propagation. Implementing DenseNet in Rust provides an opportunity to explore these innovations while taking advantage of Rust’s robust performance capabilities, making it an ideal choice for both research and industry applications.
6.6. EfficientNet and Model Scaling
EfficientNet is a family of convolutional neural network (CNN) models introduced in the paper "EfficientNet: Rethinking Model Scaling for Convolutional Neural Networks" (2019) by Mingxing Tan and Quoc V. Le. The key idea behind EfficientNet is to improve the accuracy of deep learning models while maintaining computational efficiency. Traditionally, model scaling—whether increasing depth, width, or resolution—has been performed in an ad-hoc manner, without a structured method for optimizing the trade-offs between computational cost and model performance. EfficientNet addresses this by introducing a compound scaling strategy that uniformly scales depth, width, and resolution together, based on a compound coefficient. This scaling strategy results in better model performance with fewer parameters and reduced computational costs, compared to models that scale these factors independently.
In terms of performance, EfficientNet sets a new benchmark, outperforming older models like ResNet, DenseNet, and NASNet on datasets such as ImageNet, while requiring fewer parameters and FLOPs (floating point operations). The largest model in the EfficientNet family, EfficientNet-B7, achieves near-state-of-the-art accuracy with far fewer resources compared to its counterparts. This efficiency makes EfficientNet particularly valuable for real-world applications, especially in resource-constrained environments where both accuracy and computational efficiency are critical. EfficientNet's success has inspired further research into efficient model architectures, making it a cornerstone in the evolution of deep learning models that balance high accuracy with low computational demands.
EfficientNet represents a major breakthrough in deep learning architectures by introducing a novel method called compound scaling, which balances three key dimensions of a neural network: depth, width, and resolution. Traditional CNN architectures typically scale one of these dimensions independently (e.g., increasing depth or width), which can lead to inefficient models that are either too large or fail to leverage computational resources effectively. EfficientNet addresses this by proposing a compound scaling method that scales all three dimensions in a balanced way, leading to more efficient models that achieve state-of-the-art performance while using fewer parameters and less computational power.
At the heart of EfficientNet's scaling method is the compound coefficient $\phi$, which determines how much the network's depth, width, and input resolution should be scaled. Formally, the scaling for each dimension is defined as follows:
$$ \text{depth scale} = \alpha^\phi, \quad \text{width scale} = \beta^\phi, \quad \text{resolution scale} = \gamma^\phi $$
Here, $\alpha$, $\beta$, and $\gamma$ are constants that control the rate of scaling for depth, width, and resolution, respectively, while $\phi$ is the compound scaling factor. These constants are chosen such that:
$$ \alpha \cdot \beta^2 \cdot \gamma^2 \approx 2 $$
This constraint ensures that the model grows in a balanced way, doubling the computational cost while maintaining a balanced increase in all three dimensions. In this way, EfficientNet avoids over-expanding one dimension at the expense of others, leading to a more efficient network.
EfficientNet is a state-of-the-art convolutional neural network (CNN) architecture that introduces a novel building block known as the Mobile Inverted Bottleneck Convolution (MBConv). Inspired by MobileNetV2’s depthwise separable convolutions, MBConv enhances computational efficiency while preserving representational power. It achieves this by using an expansion layer to increase the number of channels, followed by depthwise convolutions and a projection layer to reduce the channels back down. This structure reduces the computational load compared to traditional convolutions, allowing for more efficient use of resources without sacrificing accuracy. The EfficientNet family includes models from EfficientNet-B0, the baseline, to EfficientNet-B7, with each successive model scaling up in terms of depth, width, and input resolution. The key innovation behind EfficientNet is its compound scaling method, which applies a balanced scaling of depth, width, and resolution to achieve better performance without the computational explosion typically seen when scaling networks in a traditional way.
The compound scaling strategy in EfficientNet enables the models to outperform other architectures with similar parameter counts by scaling all three dimensions (depth, width, and resolution) proportionally. The baseline model, EfficientNet-B0, is optimized through a process known as Neural Architecture Search (NAS), which automates the discovery of the best architecture for a given task. NAS searches through a vast space of possible architectures to find the most efficient design. Once the optimal baseline model is discovered, the compound scaling method is applied to create larger versions of the model, from EfficientNet-B1 to EfficientNet-B7, which increasingly scale up the network's depth, width, and input resolution. This results in models that deliver high performance across a wide range of applications, from mobile devices with limited computational power (EfficientNet-B0 or B1) to large-scale server environments where accuracy is paramount (EfficientNet-B7).
EfficientNet’s approach to scaling and NAS offers several advantages over traditional methods. In conventional networks, increasing the depth or width of a model can improve performance, but often at the cost of increased computational complexity and diminishing returns. EfficientNet’s compound scaling method addresses this issue by scaling the network in a balanced manner, ensuring that the model grows efficiently and remains computationally feasible. This makes EfficientNet one of the most resource-efficient architectures, offering excellent performance even with fewer parameters compared to other models like ResNet or DenseNet. Furthermore, this flexibility allows practitioners to choose a version of EfficientNet that best fits their specific use case, whether it's for high-efficiency mobile applications or high-accuracy server-based deployments. The combination of NAS and compound scaling is what enables EfficientNet to achieve superior accuracy and efficiency, making it a versatile and high-performing model for both research and real-world applications.
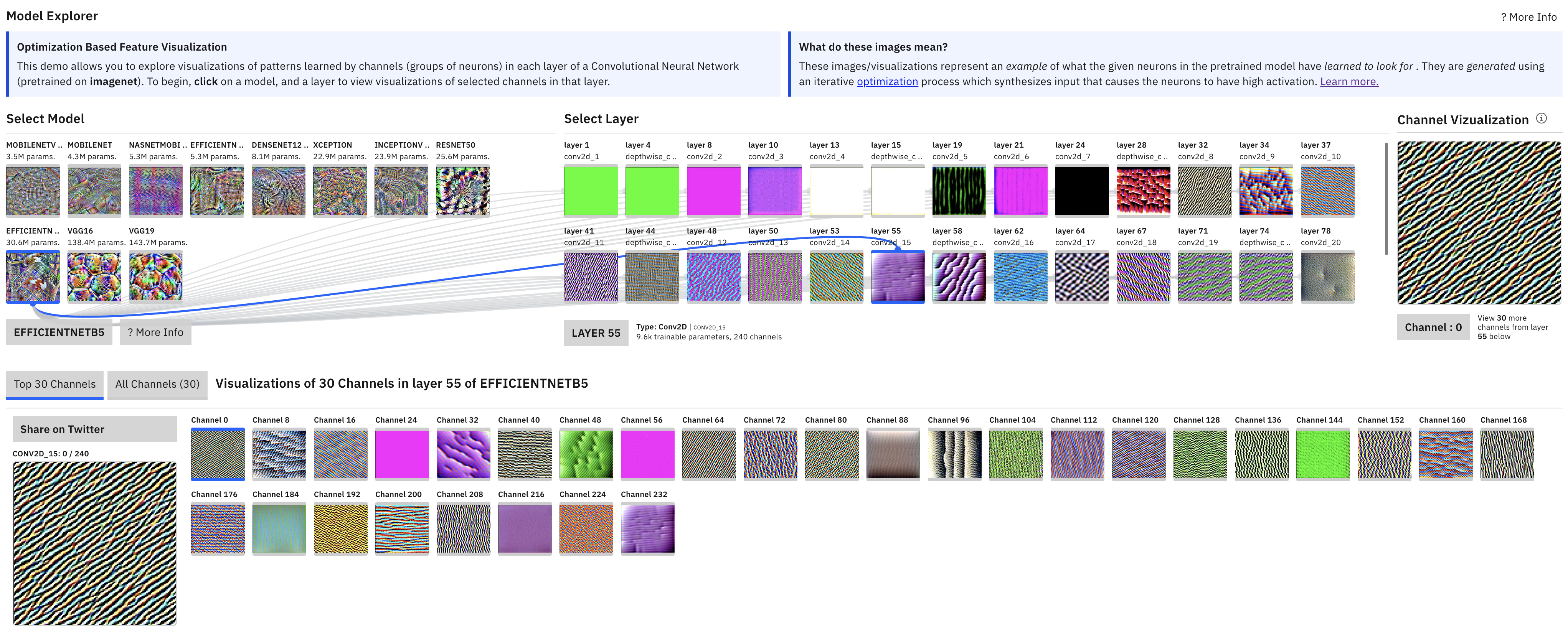
Figure 6: Visualization of EfficientNet-B5 architecture.
This Rust program demonstrates how to load and run an EfficientNet model for image classification using the candle
deep learning framework. The model variants range from EfficientNet-B0 to EfficientNet-B7, each offering different trade-offs between computational efficiency and model accuracy. The program supports loading pre-trained model weights from the Hugging Face Hub or from a local file if specified by the user. The input image, typically of size 224x224 pixels, is loaded, pre-processed, and passed through the selected EfficientNet model to predict the top 5 classes along with their probabilities. The program outputs the predictions in a human-readable format, showing the class names and their corresponding confidence scores.
//! EfficientNet implementation.
//!
//! https://arxiv.org/abs/1905.11946
use candle_core::{DType, IndexOp, D};
use candle_nn::{Module, VarBuilder};
use candle_transformers::models::efficientnet::{EfficientNet, MBConvConfig};
use clap::{Parser, ValueEnum};
use hf_hub::api::sync::Api; // Ensure we use the correct API for model downloading
#[derive(Clone, Copy, Debug, ValueEnum)]
enum Which {
B0,
B1,
B2,
B3,
B4,
B5,
B6,
B7,
}
#[derive(Parser)]
struct Args {
#[arg(long)]
model: Option<String>,
#[arg(long)]
image: String,
/// Run on CPU rather than on GPU.
#[arg(long)]
cpu: bool,
/// Variant of the model to use.
#[arg(value_enum, long, default_value_t = Which::B2)]
which: Which,
}
pub fn main() -> anyhow::Result<()> {
let args = Args::parse();
// Select device based on whether CPU or GPU is requested
let device = candle_examples::device(args.cpu)?;
// Load and prepare the image for the model (224x224 for EfficientNet)
let image = candle_examples::imagenet::load_image224(args.image)?.to_device(&device)?;
println!("Loaded image: {image:?}");
// Download the model if not specified
let model_file = match args.model {
None => {
let api = Api::new()?;
let api = api.model("lmz/candle-efficientnet".into());
let filename = match args.which {
Which::B0 => "efficientnet-b0.safetensors",
Which::B1 => "efficientnet-b1.safetensors",
Which::B2 => "efficientnet-b2.safetensors",
Which::B3 => "efficientnet-b3.safetensors",
Which::B4 => "efficientnet-b4.safetensors",
Which::B5 => "efficientnet-b5.safetensors",
Which::B6 => "efficientnet-b6.safetensors",
Which::B7 => "efficientnet-b7.safetensors",
};
api.get(filename)?
}
Some(model) => model.into(),
};
// Load the model weights into memory
let vb = unsafe { VarBuilder::from_mmaped_safetensors(&[model_file], DType::F32, &device)? };
// Set up the EfficientNet configuration based on the selected variant
let cfg = match args.which {
Which::B0 => MBConvConfig::b0(),
Which::B1 => MBConvConfig::b1(),
Which::B2 => MBConvConfig::b2(),
Which::B3 => MBConvConfig::b3(),
Which::B4 => MBConvConfig::b4(),
Which::B5 => MBConvConfig::b5(),
Which::B6 => MBConvConfig::b6(),
Which::B7 => MBConvConfig::b7(),
};
// Instantiate the EfficientNet model
let model = EfficientNet::new(vb, cfg, candle_examples::imagenet::CLASS_COUNT as usize)?;
println!("Model built successfully");
// Run inference on the input image
let logits = model.forward(&image.unsqueeze(0)?)?;
// Apply softmax to the logits to get the probabilities
let prs = candle_nn::ops::softmax(&logits, D::Minus1)?
.i(0)?
.to_vec1::<f32>()?;
// Sort the predictions by probability in descending order
let mut prs = prs.iter().enumerate().collect::<Vec<_>>();
prs.sort_by(|(_, p1), (_, p2)| p2.total_cmp(p1));
// Print top 5 predicted categories with their probabilities
for &(category_idx, pr) in prs.iter().take(5) {
println!(
"{:24}: {:.2}%",
candle_examples::imagenet::CLASSES[category_idx],
100. * pr
);
}
Ok(())
}
The code begins by parsing command-line arguments to determine the model variant (B0
to B7
), the input image, and whether the computation should run on a CPU or GPU. It then loads the image, resizes it to 224x224 pixels, and moves it to the appropriate device (CPU or GPU). The model weights are loaded either from a local file or downloaded from the Hugging Face Hub. The EfficientNet::new()
function is used to initialize the model with the selected configuration, and the model is set up based on the chosen variant (such as B0, B1, etc.). The image is passed through the model for inference, and the logits (raw output) are converted to probabilities using the softmax function. Finally, the program sorts and prints the top 5 predicted categories along with their associated probabilities. This structure ensures the efficient use of memory and computation while achieving high performance for image classification tasks.
EfficientNet’s compound scaling revolutionizes the design of neural networks by introducing a systematic method to balance depth, width, and resolution for optimizing model performance and computational cost. This scalability enables developers to train different versions of EfficientNet, from B0 to B7, to meet a variety of application needs. EfficientNet-B0, for instance, is compact and efficient, making it ideal for mobile and embedded systems, while EfficientNet-B7 offers state-of-the-art accuracy for large-scale image classification tasks, albeit at higher computational demands. By tuning scaling factors, practitioners can optimize the trade-off between accuracy and resource consumption, ensuring that EfficientNet can be effectively applied across a wide range of real-world use cases, including image classification, object detection, and medical imaging. Its adaptability has made EfficientNet a popular choice in diverse industries, where both performance and efficiency are critical. Compact models like EfficientNet-B0 and B1 are preferred for mobile applications due to their low latency, while larger models like EfficientNet-B7 are better suited for server-based deployments where computational resources are abundant.
MobileNetV4, introduced as a universal model for the mobile ecosystem, represents a significant step forward in designing lightweight neural networks for resource-constrained environments. Building on the success of its predecessors, MobileNetV4 incorporates Mobile Neural Architecture Search (NAS), an automated process that optimizes model design for specific tasks, allowing it to achieve high accuracy with minimal computational resources. Leveraging lightweight operations like depthwise separable convolutions, MobileNetV4 minimizes the number of operations required for inference, making it particularly suitable for devices with limited processing power such as smartphones and IoT devices. Furthermore, MobileNetV4 introduces the concept of universal models that can adapt to both mobile and cloud environments, offering scalability across devices ranging from edge systems to high-performance servers. Its ability to deliver real-time performance for tasks like object detection, image classification, and augmented reality applications highlights its versatility and efficiency in addressing modern AI challenges.
MobileNetV4 is a cutting-edge neural network architecture specifically designed to cater to mobile and resource-constrained environments. Building on the principles of previous MobileNet versions, MobileNetV4 emphasizes efficiency and flexibility, making it ideal for a wide range of applications, including image classification, object detection, and real-time processing on devices with limited computational power. Key innovations include lightweight operations such as depthwise separable convolutions and enhancements through Neural Architecture Search (NAS), allowing MobileNetV4 to balance accuracy and computational efficiency. This architecture offers multiple variants, such as Small, Medium, and Large, tailored to specific use cases, with hybrid configurations providing additional adaptability for diverse computational needs. Its ability to scale across devices, from low-power edge devices to more capable systems, highlights its versatility and robustness in modern machine learning applications.
use accelerate_src;
use clap::{Parser, ValueEnum};
use candle::{DType, IndexOp, D};
use candle_nn::{Module, VarBuilder};
use candle_transformers::models::mobilenetv4;
#[derive(Clone, Copy, Debug, ValueEnum)]
enum Which {
Small,
Medium,
Large,
HybridMedium,
HybridLarge,
}
impl Which {
fn model_filename(&self) -> String {
let name = match self {
Self::Small => "conv_small.e2400_r224",
Self::Medium => "conv_medium.e500_r256",
Self::HybridMedium => "hybrid_medium.ix_e550_r256",
Self::Large => "conv_large.e600_r384",
Self::HybridLarge => "hybrid_large.ix_e600_r384",
};
format!("timm/mobilenetv4_{}_in1k", name)
}
fn resolution(&self) -> u32 {
match self {
Self::Small => 224,
Self::Medium => 256,
Self::HybridMedium => 256,
Self::Large => 384,
Self::HybridLarge => 384,
}
}
fn config(&self) -> mobilenetv4::Config {
match self {
Self::Small => mobilenetv4::Config::small(),
Self::Medium => mobilenetv4::Config::medium(),
Self::HybridMedium => mobilenetv4::Config::hybrid_medium(),
Self::Large => mobilenetv4::Config::large(),
Self::HybridLarge => mobilenetv4::Config::hybrid_large(),
}
}
}
#[derive(Parser)]
struct Args {
#[arg(long)]
model: Option<String>,
#[arg(long)]
image: String,
/// Run on CPU rather than on GPU.
#[arg(long)]
cpu: bool,
#[arg(value_enum, long, default_value_t=Which::Small)]
which: Which,
}
pub fn main() -> anyhow::Result<()> {
let args = Args::parse();
let device = candle_examples::device(args.cpu)?;
let image =
candle_examples::imagenet::load_image(args.image, args.which.resolution() as usize)?
.to_device(&device)?;
println!("loaded image {image:?}");
let model_file = match args.model {
None => {
let model_name = args.which.model_filename();
let api = hf_hub::api::sync::Api::new()?;
let api = api.model(model_name);
api.get("model.safetensors")?
}
Some(model) => model.into(),
};
let vb = unsafe { VarBuilder::from_mmaped_safetensors(&[model_file], DType::F32, &device)? };
let model = mobilenetv4::mobilenetv4(&args.which.config(), 1000, vb)?;
println!("model built");
let logits = model.forward(&image.unsqueeze(0)?)?;
let prs = candle_nn::ops::softmax(&logits, D::Minus1)?
.i(0)?
.to_vec1::<f32>()?;
let mut prs = prs.iter().enumerate().collect::<Vec<_>>();
prs.sort_by(|(_, p1), (_, p2)| p2.total_cmp(p1));
for &(category_idx, pr) in prs.iter().take(5) {
println!(
"{:24}: {:.2}%",
candle_examples::imagenet::CLASSES[category_idx],
100. * pr
);
}
Ok(())
}
The provided code implements MobileNetV4 using the Rust-based candle
deep learning framework. It allows users to select a MobileNetV4 variant (e.g., Small, Medium, Large) via command-line arguments and dynamically adjusts the model configuration and image preprocessing resolution accordingly. The code supports model loading either from a local file or by downloading pre-trained weights from the Hugging Face Hub. Once the model is initialized, it preprocesses an input image to match the resolution expected by the selected variant, performs inference, and applies softmax to convert logits into class probabilities. Finally, it sorts and displays the top 5 predictions along with their confidence scores, offering an efficient and flexible pipeline for leveraging MobileNetV4 in image classification tasks. This implementation highlights the model’s adaptability and computational efficiency, making it well-suited for various environments.
Together, EfficientNet and MobileNetV4 represent a transformative approach to neural network design, each catering to the growing need for efficient and scalable architectures. EfficientNet excels in environments requiring a balance between high accuracy and computational efficiency through its compound scaling strategy, making it versatile across industries and applications. MobileNetV4, on the other hand, prioritizes lightweight, real-time inference with its NAS-driven architecture and universal applicability, excelling in mobile and embedded systems. Both models share a common goal of optimizing neural networks for diverse computational environments, from low-power edge devices to large-scale cloud computing platforms. By implementing these architectures in Rust, developers can capitalize on Rust’s performance and safety features, further enhancing the efficiency and reliability of AI systems. Together, EfficientNet and MobileNetV4 exemplify the cutting-edge of machine learning innovation, driving the development of next-generation applications with unparalleled scalability and resource efficiency.
6.7. YOLO Models and Industry Practices
The YOLO (You Only Look Once) model represents a breakthrough in the field of computer vision, particularly in object detection. The development of YOLO was driven by the need for faster and more efficient models that could simultaneously perform both object localization and classification in real-time. Prior to YOLO, the dominant approach for object detection involved region proposal networks (RPNs) and two-stage architectures like R-CNN (Regions with Convolutional Neural Networks), which were relatively slow and computationally expensive. These models first identified potential object regions, and then classified each region, resulting in a multi-step process that was not conducive to real-time applications.
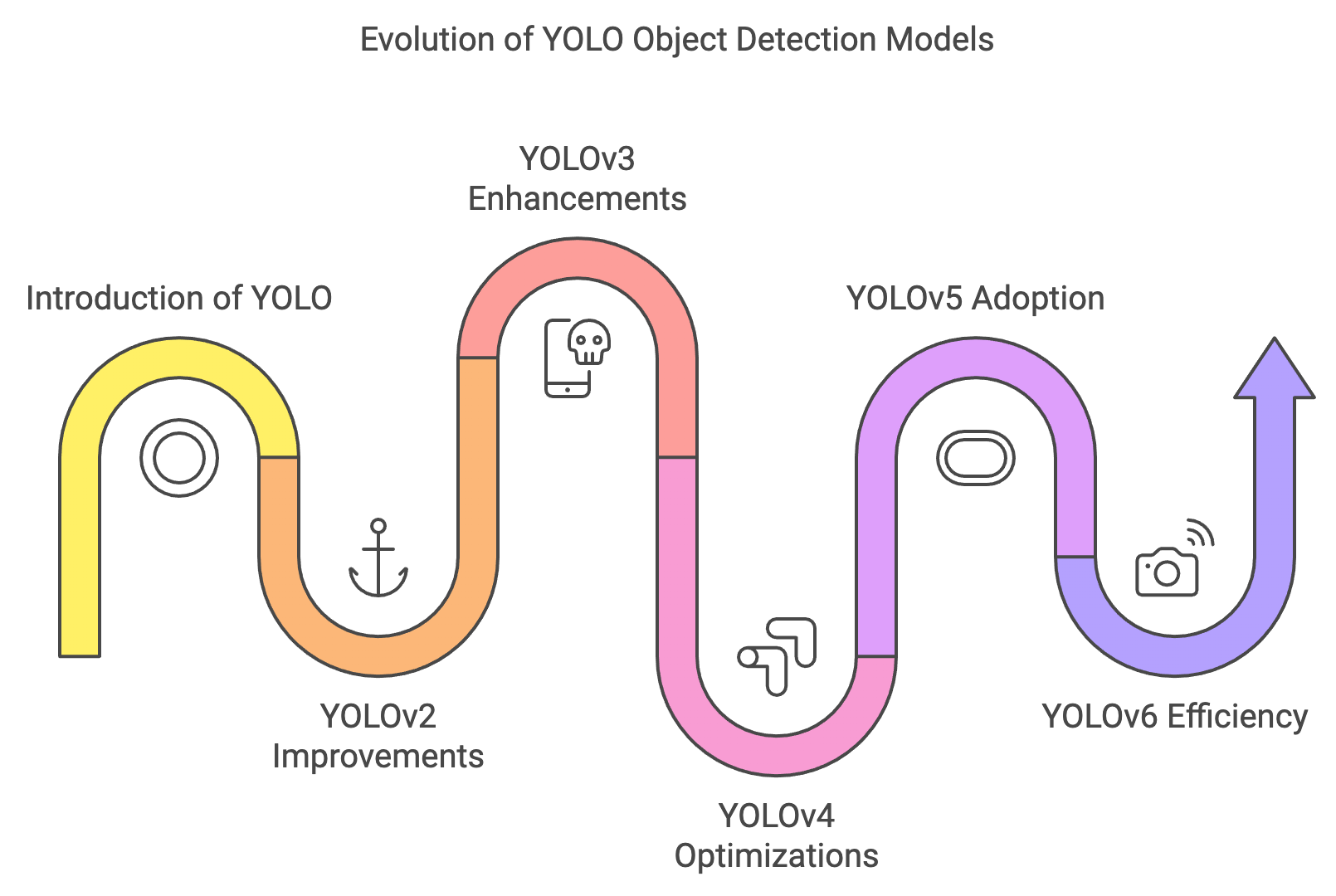
Figure 7: Historical journey of YOLO models.
The first version of YOLO, introduced by Joseph Redmon and his colleagues in 2015, was revolutionary because it unified the task of object detection into a single network, which could predict both bounding boxes and class probabilities directly from the image in one pass. This greatly improved the speed of object detection while maintaining competitive accuracy. YOLO's key feature was the concept of dividing an image into a grid and having each grid cell predict bounding boxes and class probabilities. By doing so, YOLO was able to detect objects in a single forward pass, significantly reducing computational complexity compared to previous methods.
YOLOv2, released in 2016, introduced several significant improvements. One of the major changes was the use of a deeper architecture, specifically the Darknet-19 network, which allowed for better feature extraction and higher accuracy. YOLOv2 also introduced the use of anchor boxes, which improved the model's ability to predict bounding boxes more accurately, especially for objects with different aspect ratios. Additionally, it incorporated batch normalization, which helped stabilize training and improved the model's performance. This version of YOLO marked a substantial increase in both speed and accuracy, and was able to handle a wider variety of objects more effectively.
YOLOv3, launched in 2018, further refined the model's performance. It introduced a new backbone network, Darknet-53, which was significantly more powerful than its predecessor and allowed for better detection of smaller objects. YOLOv3 also introduced multi-scale detection, where the model made predictions at three different scales. This allowed YOLOv3 to detect objects of various sizes more effectively, further improving its versatility. The use of independent logistic regression for objectness prediction and the ability to detect more fine-grained features made YOLOv3 one of the most popular models for real-time object detection tasks at the time.
YOLOv4, released in 2020, brought a series of optimizations that made the model more accurate and efficient. YOLOv4 introduced a number of advanced techniques such as cross-stage partial connections (CSP), spatial pyramid pooling (SPP), and mish activation functions. These innovations helped to improve the model’s robustness and generalization capabilities. YOLOv4 also improved the training pipeline, using techniques like multi-scale training and auto-learning bounding box anchors, which helped the model adapt better to a variety of datasets. Additionally, YOLOv4 improved the model's ability to handle large-scale datasets and achieve state-of-the-art results on benchmarks such as COCO.
The next iteration, YOLOv5, was not officially released by the original creators of YOLO, but by a separate group (Ultralytics), and it gained widespread adoption due to its simplicity and ease of use. YOLOv5 featured a more user-friendly interface and introduced features like automatic mixed-precision training, improved pre-trained weights, and better performance on edge devices. It also improved speed and accuracy through innovations like efficient convolutional layers and optimized post-processing steps. While not officially part of the original YOLO series, YOLOv5 became popular in both academic and industry settings due to its performance and accessibility.
YOLOv6, released in 2022, introduced a more efficient architecture for real-time object detection with enhanced accuracy. YOLOv6 also emphasized its ability to run efficiently on various hardware, including mobile devices and embedded systems, making it a top choice for industrial applications requiring fast processing on edge devices.
Throughout its evolution, YOLO has consistently pushed the boundaries of object detection, focusing on both speed and accuracy, making it one of the most widely used models in practical applications today. The combination of simplicity, efficiency, and powerful detection capabilities has made YOLO the go-to solution for real-time object detection in fields such as autonomous vehicles, surveillance systems, and robotics. Each version of YOLO has introduced key innovations that have allowed the model to evolve into a powerful tool for both research and industrial applications.
Here is a detailed explanation of the YOLOv3 architecture. It is a convolutional neural network (CNN) that builds upon the previous versions by enhancing feature extraction and introducing multi-scale detection capabilities. YOLOv3 uses Darknet-53 as its backbone network, a deep convolutional network designed for high-performance image classification and object detection tasks. Darknet-53 is an improvement over the previous backbone, Darknet-19, which was used in YOLOv2.
Architecture: Darknet-53 is composed of 53 convolutional layers, and it integrates residual connections, which were inspired by ResNet. These residual connections help in mitigating the vanishing gradient problem and allow the network to be deeper without losing performance. By adding these skip connections, YOLOv3 improves both training stability and model performance.
Convolutional Layers: Darknet-53 uses a mix of 3x3 and 1x1 convolutional layers. The 1x1 convolutions are used for dimensionality reduction and to increase the depth of the model, while the 3x3 convolutions are responsible for capturing high-level spatial features.
Leaky ReLU Activation: YOLOv3 uses the Leaky ReLU activation function throughout the network. This activation function helps improve the model's ability to deal with negative values by allowing a small, non-zero gradient when the unit is not active (negative side).
One of the key innovations introduced in YOLOv3 is multi-scale detection. This allows the network to predict bounding boxes at three different scales, making it more adept at detecting objects of varying sizes.
Feature Maps: YOLOv3 generates predictions at three different feature map resolutions. These feature maps correspond to different stages of the network, with the earlier layers detecting smaller objects and the deeper layers detecting larger objects. The model outputs predictions from three different layers: one after the 32nd, one after the 61st, and one after the 75th convolutional layer. These layers correspond to large, medium, and small objects, respectively.
Upsampling and Downsampling: The network uses upsampling and downsampling operations to detect objects at various scales. The upsampling helps to match the lower-resolution feature maps with the larger ones, which improves the detection of smaller objects. Conversely, downsampling allows the network to use more abstracted features for detecting larger objects.
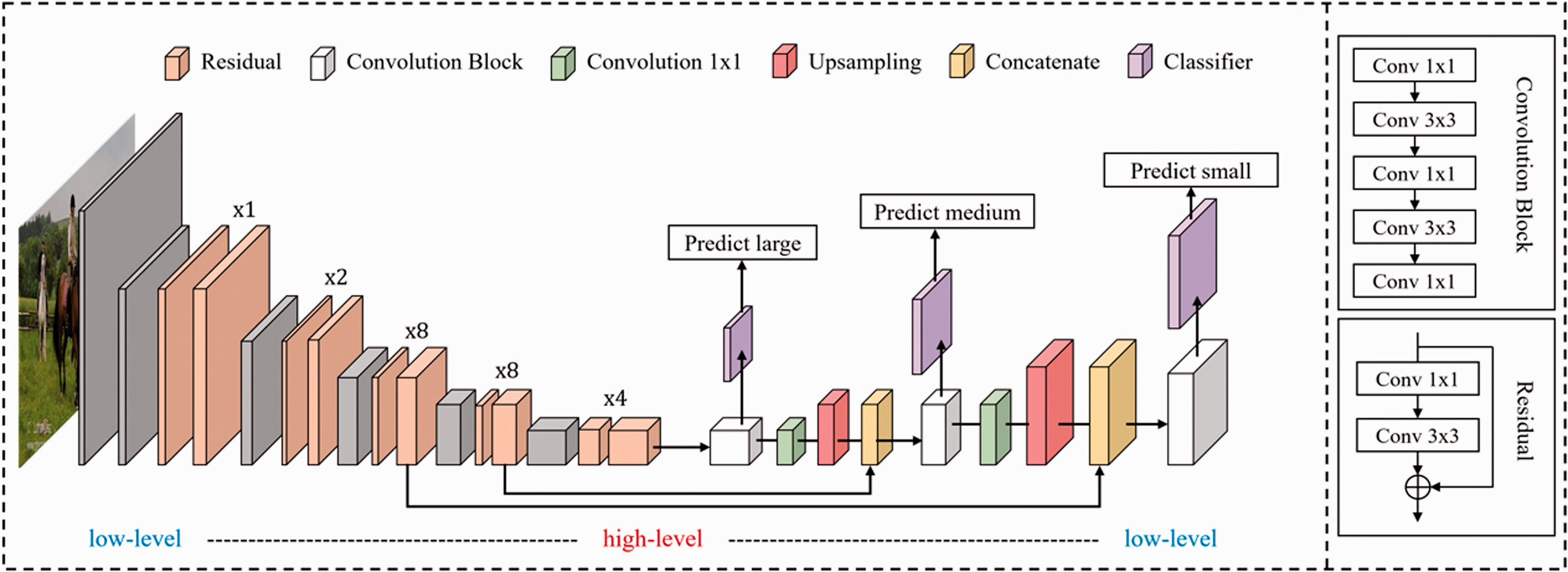
Figure 8: YOLO 3 architecture with duplex FPN.
YOLOv3 introduces the concept of anchor boxes, which helps improve the accuracy of bounding box predictions. In YOLOv3, each grid cell predicts multiple bounding boxes using predefined anchor boxes.
Bounding Box Predictions: For each grid cell, YOLOv3 predicts a total of three bounding boxes. Each bounding box prediction includes four values: the coordinates of the box (center x and y, width, and height) relative to the grid cell, the objectness score (which measures the confidence that the box contains an object), and the class probabilities (which indicate the likelihood of the object belonging to each of the possible classes).
Anchor Boxes: YOLOv3 uses pre-defined anchor boxes, which are a set of representative bounding box shapes derived from the dataset (usually using k-means clustering). These anchor boxes allow YOLOv3 to better predict bounding boxes that match the shapes and aspect ratios of objects in the dataset. By predicting multiple bounding boxes per grid cell, YOLOv3 can more accurately capture the diversity of object shapes and sizes.
The YOLOv3 loss function is a combination of several components that guide the model during training.
Localization Loss: This component is responsible for penalizing incorrect bounding box predictions. The localization loss is computed using the mean squared error between the predicted and ground truth bounding box coordinates (center x, center y, width, and height).
Confidence Loss: This loss penalizes the network for incorrect objectness predictions. It is based on the intersection over union (IoU) between the predicted and ground truth bounding boxes. When there is an object present, the model tries to minimize the difference between its predicted objectness score and the ground truth (1 for an object, 0 for background).
Class Prediction Loss: YOLOv3 uses binary cross-entropy loss to calculate the error between the predicted class probabilities and the ground truth. This loss penalizes the network for incorrect object classification.
The final loss is a weighted sum of these individual losses, which is used to update the model’s weights during training. YOLOv3 outputs a tensor that contains predictions for each grid cell in the image. The tensor has a shape of (S x S x (B * 5 + C))
, where:
S x S represents the grid size (e.g., 13x13 for a 416x416 input image),
B is the number of bounding boxes predicted per grid cell (in YOLOv3, B = 3),
5 represents the number of parameters for each bounding box (center x, center y, width, height, and objectness score),
C is the number of classes.
For each grid cell, YOLOv3 predicts the following values:
Three sets of bounding box predictions (with 4 coordinates each),
Three objectness scores (one for each bounding box),
Class probabilities for each of the C classes.
After YOLOv3 generates bounding box predictions, it performs non-maximum suppression (NMS) to filter out redundant and overlapping boxes. NMS is used to select the best bounding box for each object by removing boxes with lower objectness scores and those that overlap too much with higher-scoring boxes (based on a threshold for the IoU).
In summary, YOLOv3 improves on its predecessors by introducing a more powerful backbone network (Darknet-53), multi-scale detection, and anchor box predictions. The architecture is designed to be fast, accurate, and scalable for a wide range of object detection tasks. By predicting bounding boxes at multiple scales and using a more sophisticated loss function, YOLOv3 is able to achieve a good balance between speed and accuracy, making it one of the most widely used object detection models in both research and industry applications.
The YOLOv3 configuration file below (yolo-v3.cfg) defines the architecture and training parameters for the model. The [net]
section contains critical training settings, such as batch size, subdivisions, input image dimensions (416x416), and data augmentation parameters like hue, exposure, and saturation. These configurations affect the training process, helping the model generalize better by simulating various image conditions. The learning rate and optimization settings, including the momentum and decay parameters, as well as the learning rate scheduling policy (steps
), control how the model’s weights are updated during training. The max_batches
parameter defines the total number of training iterations, while the burn_in
parameter specifies the number of iterations before the learning rate reaches its initial value.
[net]
# Testing
batch=1
subdivisions=1
# Training
# batch=64
# subdivisions=16
width= 416
height = 416
channels=3
momentum=0.9
decay=0.0005
angle=0
saturation = 1.5
exposure = 1.5
hue=.1
learning_rate=0.001
burn_in=1000
max_batches = 500200
policy=steps
steps=400000,450000
scales=.1,.1
[convolutional]
batch_normalize=1
filters=32
size=3
stride=1
pad=1
activation=leaky
# Downsample
[convolutional]
batch_normalize=1
filters=64
size=3
stride=2
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=32
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=64
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
# Downsample
[convolutional]
batch_normalize=1
filters=128
size=3
stride=2
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=64
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=128
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
[convolutional]
batch_normalize=1
filters=64
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=128
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
# Downsample
[convolutional]
batch_normalize=1
filters=256
size=3
stride=2
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=128
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=256
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
[convolutional]
batch_normalize=1
filters=128
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=256
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
[convolutional]
batch_normalize=1
filters=128
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=256
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
[convolutional]
batch_normalize=1
filters=128
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=256
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
[convolutional]
batch_normalize=1
filters=128
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=256
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
[convolutional]
batch_normalize=1
filters=128
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=256
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
[convolutional]
batch_normalize=1
filters=128
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=256
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
[convolutional]
batch_normalize=1
filters=128
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=256
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
# Downsample
[convolutional]
batch_normalize=1
filters=512
size=3
stride=2
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=256
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=512
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
[convolutional]
batch_normalize=1
filters=256
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=512
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
[convolutional]
batch_normalize=1
filters=256
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=512
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
[convolutional]
batch_normalize=1
filters=256
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=512
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
[convolutional]
batch_normalize=1
filters=256
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=512
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
[convolutional]
batch_normalize=1
filters=256
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=512
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
[convolutional]
batch_normalize=1
filters=256
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=512
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
[convolutional]
batch_normalize=1
filters=256
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=512
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
# Downsample
[convolutional]
batch_normalize=1
filters=1024
size=3
stride=2
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=512
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=1024
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
[convolutional]
batch_normalize=1
filters=512
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=1024
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
[convolutional]
batch_normalize=1
filters=512
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=1024
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
[convolutional]
batch_normalize=1
filters=512
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
filters=1024
size=3
stride=1
pad=1
activation=leaky
[shortcut]
from=-3
activation=linear
######################
[convolutional]
batch_normalize=1
filters=512
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
size=3
stride=1
pad=1
filters=1024
activation=leaky
[convolutional]
batch_normalize=1
filters=512
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
size=3
stride=1
pad=1
filters=1024
activation=leaky
[convolutional]
batch_normalize=1
filters=512
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
size=3
stride=1
pad=1
filters=1024
activation=leaky
[convolutional]
size=1
stride=1
pad=1
filters=255
activation=linear
[yolo]
mask = 6,7,8
anchors = 10,13, 16,30, 33,23, 30,61, 62,45, 59,119, 116,90, 156,198, 373,326
classes=80
num=9
jitter=.3
ignore_thresh = .5
truth_thresh = 1
random=1
[route]
layers = -4
[convolutional]
batch_normalize=1
filters=256
size=1
stride=1
pad=1
activation=leaky
[upsample]
stride=2
[route]
layers = -1, 61
[convolutional]
batch_normalize=1
filters=256
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
size=3
stride=1
pad=1
filters=512
activation=leaky
[convolutional]
batch_normalize=1
filters=256
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
size=3
stride=1
pad=1
filters=512
activation=leaky
[convolutional]
batch_normalize=1
filters=256
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
size=3
stride=1
pad=1
filters=512
activation=leaky
[convolutional]
size=1
stride=1
pad=1
filters=255
activation=linear
[yolo]
mask = 3,4,5
anchors = 10,13, 16,30, 33,23, 30,61, 62,45, 59,119, 116,90, 156,198, 373,326
classes=80
num=9
jitter=.3
ignore_thresh = .5
truth_thresh = 1
random=1
[route]
layers = -4
[convolutional]
batch_normalize=1
filters=128
size=1
stride=1
pad=1
activation=leaky
[upsample]
stride=2
[route]
layers = -1, 36
[convolutional]
batch_normalize=1
filters=128
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
size=3
stride=1
pad=1
filters=256
activation=leaky
[convolutional]
batch_normalize=1
filters=128
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
size=3
stride=1
pad=1
filters=256
activation=leaky
[convolutional]
batch_normalize=1
filters=128
size=1
stride=1
pad=1
activation=leaky
[convolutional]
batch_normalize=1
size=3
stride=1
pad=1
filters=256
activation=leaky
[convolutional]
size=1
stride=1
pad=1
filters=255
activation=linear
[yolo]
mask = 0,1,2
anchors = 10,13, 16,30, 33,23, 30,61, 62,45, 59,119, 116,90, 156,198, 373,326
classes=80
num=9
jitter=.3
ignore_thresh = .5
truth_thresh = 1
random=1
The core of the model is built using a series of convolutional layers that extract features at different scales. Each convolutional layer is followed by batch normalization to stabilize learning and Leaky ReLU activation functions to introduce non-linearity. The model also employs shortcut connections (from earlier layers) to enhance feature propagation and help mitigate the vanishing gradient problem. This is especially important in deeper layers to maintain high performance while avoiding the degradation that can occur with deeper networks. The network progressively downsamples the image through convolutional layers with stride 2 to reduce spatial dimensions, while increasing the number of filters to capture more complex features. These downsampled feature maps are critical for detecting smaller objects in higher-resolution layers.
The configuration also defines several key architectural components, such as the use of 1x1 convolutions for reducing the number of channels and 3x3 convolutions for expanding the feature maps. The use of residual connections, or "shortcuts," between layers ensures that the information is passed directly between distant layers, allowing the model to learn more efficiently and with greater accuracy. Finally, the model ends with convolutional layers designed to output prediction values: the objectness score, bounding box coordinates, and class probabilities. These predictions are made at different scales throughout the network, with each scale responsible for detecting objects of varying sizes. The network is designed to predict bounding boxes at three different levels of resolution to improve detection accuracy for small, medium, and large objects.
The model's configuration file (yolo-v3.cfg) enables flexible training and fine-tuning by adjusting batch sizes, learning rates, and augmentation parameters to suit different datasets and tasks. The overall design focuses on balancing computational efficiency and detection accuracy, making YOLOv3 suitable for real-time object detection applications.
Given the configuration file, the code below defines a basic structure for loading a YOLOv3 model configuration, parsing it, and performing inference on input images using the Rust programming language. The main components include the Darknet
struct, which represents the YOLO model, and the Block
struct, which represents individual sections of the model configuration. The Darknet
struct contains methods for reading the configuration file (parse_config
), defining the model structure (build_model
), and retrieving the model's input size (width
and height
). The configuration file, in this case, yolo-v3.cfg
, defines the layers and parameters for building the model. The main
function initializes the neural network using the configuration and loads pretrained weights from the file specified by the user. It then processes each image provided, resizing them to fit the model's input size, performing forward passes through the model, and printing the results.
[dependencies]
anyhow = "1.0"
tch = "0.12"
reqwest = { version = "0.11", features = ["blocking"] }
flate2 = "1.0"
tokio = { version = "1", features = ["full"] }
tar = "0.4.43"
rand = "0.8.5"
use anyhow::{ensure, Result};
use std::collections::BTreeMap;
use std::fs::File;
use std::io::{BufRead, BufReader};
use std::path::Path;
use tch::nn::{self, ModuleT};
use tch::vision::image;
// Constants
const CONFIG_NAME: &str = "examples/yolo/yolo-v3.cfg";
// COCO class names
pub const NAMES: [&str; 80] = [
"person", "bicycle", "car", "motorbike", "aeroplane", "bus", "train", "truck", "boat",
"traffic light", "fire hydrant", "stop sign", "parking meter", "bench", "bird", "cat", "dog",
"horse", "sheep", "cow", "elephant", "bear", "zebra", "giraffe", "backpack", "umbrella",
"handbag", "tie", "suitcase", "frisbee", "skis", "snowboard", "sports ball", "kite",
"baseball bat", "baseball glove", "skateboard", "surfboard", "tennis racket", "bottle",
"wine glass", "cup", "fork", "knife", "spoon", "bowl", "banana", "apple", "sandwich",
"orange", "broccoli", "carrot", "hot dog", "pizza", "donut", "cake", "chair", "sofa",
"pottedplant", "bed", "diningtable", "toilet", "tvmonitor", "laptop", "mouse", "remote",
"keyboard", "cell phone", "microwave", "oven", "toaster", "sink", "refrigerator", "book",
"clock", "vase", "scissors", "teddy bear", "hair drier", "toothbrush",
];
#[allow(dead_code)] // Suppress warnings for unused fields
pub struct Darknet {
blocks: Vec<Block>,
parameters: BTreeMap<String, String>,
}
impl Darknet {
pub fn parse_config<T: AsRef<Path>>(path: T) -> Result<Self> {
let file = File::open(path)?;
let mut blocks = vec![];
let mut current_block = None;
for line in BufReader::new(file).lines() {
let line = line?;
if line.starts_with('[') {
if let Some(block) = current_block.take() {
blocks.push(block);
}
current_block = Some(Block::new(&line[1..line.len() - 1])?);
} else if let Some(block) = current_block.as_mut() {
block.add_parameter(&line)?;
}
}
if let Some(block) = current_block {
blocks.push(block);
}
Ok(Self {
blocks,
parameters: BTreeMap::new(),
})
}
pub fn build_model(&self, _vs: &nn::Path) -> Result<nn::FuncT> {
// Placeholder implementation
unimplemented!(); // Replace with actual YOLO model construction
}
pub fn width(&self) -> Result<i64> {
Ok(416) // Example: default YOLO width
}
pub fn height(&self) -> Result<i64> {
Ok(416) // Example: default YOLO height
}
}
#[allow(dead_code)] // Suppress warnings for unused fields
pub struct Block {
block_type: String,
parameters: BTreeMap<String, String>,
}
impl Block {
pub fn new(block_type: &str) -> Result<Self> {
Ok(Self {
block_type: block_type.to_string(),
parameters: BTreeMap::new(),
})
}
pub fn add_parameter(&mut self, line: &str) -> Result<()> {
if let Some((key, value)) = line.split_once('=') {
self.parameters
.insert(key.trim().to_string(), value.trim().to_string());
}
Ok(())
}
}
// Main function
pub fn main() -> Result<()> {
let args: Vec<_> = std::env::args().collect();
ensure!(args.len() >= 3, "usage: main yolo-v3.ot img.jpg ...");
let mut vs = nn::VarStore::new(tch::Device::Cpu);
let darknet = Darknet::parse_config(CONFIG_NAME)?;
let model = darknet.build_model(&vs.root())?;
vs.load(&args[1])?;
for (index, image) in args.iter().skip(2).enumerate() {
let original_image = image::load(image)?;
let net_width = darknet.width()?;
let net_height = darknet.height()?;
let image = image::resize(&original_image, net_width, net_height)?;
let image = image.unsqueeze(0).to_kind(tch::Kind::Float) / 255.;
let _predictions = model.forward_t(&image, false).squeeze(); // Corrected: Added underscore
println!("Processed image {index}");
}
Ok(())
}
The code begins by reading the configuration file using the Darknet::parse_config
function, which processes each block (e.g., convolutional layers) and stores its parameters. The Block
struct helps manage these blocks and their associated parameters. Once the model configuration is parsed, the model structure is supposed to be constructed in build_model
, though this part is left as a placeholder and needs implementation. The main
function handles loading a pretrained YOLO model's weights, reading input images, resizing them to the required dimensions, normalizing the pixel values, and performing inference using the loaded model. The result of the forward pass (predictions) is then computed, though it is currently not fully utilized in this version of the code. This serves as a foundation for further development of a complete YOLOv3 object detection pipeline.
This Rust code provides the foundation for parsing a Darknet configuration file (used by neural networks like YOLO), extracting parameters for each layer, and constructing a neural network based on those parameters. The code utilizes the candle
and candle_nn
crates for building neural network layers such as convolutions, upsampling, and custom operations like routing, shortcuts, and YOLO detection layers. It reads the configuration file, organizes blocks of parameters into appropriate structures, and uses those blocks to build the network step-by-step. The implementation focuses on defining and interpreting YOLO model components and the necessary functions to process network layers efficiently.
[dependencies]
accelerate-src = "0.3.2"
anyhow = "1.0"
candle-core = "0.8.0"
candle-examples = "0.8.0"
candle-nn = "0.8.0"
candle-transformers = "0.8.0"
clap = { version = "4", features = ["derive"] }
hf-hub = "0.3.2"
image = "0.25.5"
use candle_core::{Result, Error}; // Import essential types for result handling and error reporting.
use candle_nn::{batch_norm, conv2d, conv2d_no_bias, Func, Module, VarBuilder}; // Import utilities for building neural network components.
use std::collections::BTreeMap; // For storing parameters as key-value pairs.
use std::fs::File; // For file handling.
use std::io::{BufRead, BufReader}; // For reading the configuration file line by line.
use std::path::Path; // For handling file paths.
/// Represents a block in the Darknet configuration file.
/// Each block has a type (e.g., "convolutional", "yolo") and a set of parameters.
#[derive(Debug)]
#[allow(dead_code)]
struct Block {
block_type: String, // The type of block (e.g., "convolutional").
parameters: BTreeMap<String, String>, // Parameters for the block as key-value pairs.
}
impl Block {
/// Retrieves the value of a parameter by its key.
/// Returns an error if the key does not exist.
#[allow(dead_code)]
fn get(&self, key: &str) -> Result<&str> {
self.parameters
.get(key) // Try to get the key from the parameters.
.ok_or_else(|| Error::Msg(format!("cannot find {} in {}", key, self.block_type))) // Error if not found.
.map(|x| x.as_str()) // Convert &String to &str.
}
}
/// Represents the entire Darknet configuration, which includes a series of blocks.
#[derive(Debug)]
pub struct Darknet {
blocks: Vec<Block>, // List of blocks parsed from the configuration file.
parameters: BTreeMap<String, String>, // Global parameters defined in the "net" block.
}
impl Darknet {
/// Retrieves the value of a global parameter by its key.
/// Returns an error if the key does not exist.
#[allow(dead_code)]
fn get(&self, key: &str) -> Result<&str> {
self.parameters
.get(key) // Try to get the key from global parameters.
.ok_or_else(|| Error::Msg(format!("cannot find {} in net parameters", key))) // Error if not found.
.map(|x| x.as_str()) // Convert &String to &str.
}
}
/// Accumulates data while parsing the configuration file.
/// Helps in grouping parameters into blocks.
struct Accumulator {
block_type: Option<String>, // The type of the current block being parsed.
parameters: BTreeMap<String, String>, // Parameters for the current block.
net: Darknet, // The resulting Darknet configuration.
}
impl Accumulator {
/// Creates a new accumulator for parsing.
fn new() -> Accumulator {
Accumulator {
block_type: None,
parameters: BTreeMap::new(),
net: Darknet {
blocks: vec![],
parameters: BTreeMap::new(),
},
}
}
/// Finalizes the current block and adds it to the Darknet configuration.
/// Clears the parameters for the next block.
fn finish_block(&mut self) {
if let Some(block_type) = &self.block_type {
if block_type == "net" {
// Global "net" block parameters are stored separately.
self.net.parameters = self.parameters.clone();
} else {
// Add other blocks to the list.
let block = Block {
block_type: block_type.to_string(),
parameters: self.parameters.clone(),
};
self.net.blocks.push(block);
}
self.parameters.clear(); // Clear parameters for the next block.
}
self.block_type = None; // Reset the block type.
}
}
/// Parses a Darknet configuration file and constructs a `Darknet` object.
pub fn parse_config<T: AsRef<Path>>(path: T) -> Result<Darknet> {
let file = File::open(path.as_ref())?; // Open the file.
let mut acc = Accumulator::new(); // Create a new accumulator.
for line in BufReader::new(file).lines() {
let line = line?;
if line.is_empty() || line.starts_with('#') {
continue; // Skip empty lines and comments.
}
let line = line.trim();
if line.starts_with('[') {
// A new block starts.
if !line.ends_with(']') {
return Err(Error::Msg(format!("line does not end with ']' {line}")));
}
acc.finish_block(); // Finalize the previous block.
acc.block_type = Some(line[1..line.len() - 1].to_string()); // Set the new block type.
} else {
// Parse key-value pairs.
let key_value: Vec<&str> = line.splitn(2, '=').collect();
if key_value.len() != 2 {
return Err(Error::Msg(format!("missing equal {line}")));
}
let prev = acc.parameters.insert(
key_value[0].trim().to_owned(),
key_value[1].trim().to_owned(),
);
if prev.is_some() {
return Err(Error::Msg(format!("multiple values for key {}", line)));
}
}
}
acc.finish_block(); // Finalize the last block.
Ok(acc.net) // Return the parsed Darknet configuration.
}
/// Represents a layer or operation in the Darknet model.
#[allow(dead_code)]
enum Bl {
Layer(Box<dyn Module + Send + Sync>), // A standard layer.
Route(Vec<usize>), // A route layer combining outputs from other layers.
Shortcut(usize), // A shortcut layer (e.g., residual connection).
Yolo(usize, Vec<(usize, usize)>), // A YOLO detection layer.
}
/// Builds a convolutional layer based on the configuration block.
#[allow(dead_code)]
fn conv(vb: VarBuilder, index: usize, p: usize, b: &Block) -> Result<(usize, Bl)> {
let activation = b.get("activation")?;
let filters = b.get("filters")?.parse::<usize>()?;
let pad = b.get("pad")?.parse::<usize>()?;
let size = b.get("size")?.parse::<usize>()?;
let stride = b.get("stride")?.parse::<usize>()?;
let padding = if pad != 0 { (size - 1) / 2 } else { 0 };
let (bn, bias) = match b.parameters.get("batch_normalize") {
Some(p) if p.parse::<usize>()? != 0 => {
let bn = batch_norm(filters, 1e-5, vb.pp(format!("batch_norm_{index}")))?;
(Some(bn), false)
}
_ => (None, true),
};
let conv_cfg = candle_nn::Conv2dConfig {
stride,
padding,
groups: 1,
dilation: 1,
};
let conv = if bias {
conv2d(p, filters, size, conv_cfg, vb.pp(format!("conv_{index}")))?
} else {
conv2d_no_bias(p, filters, size, conv_cfg, vb.pp(format!("conv_{index}")))?
};
let leaky = match activation {
"leaky" => true,
"linear" => false,
_ => return Err(Error::Msg(format!("unsupported activation {}", activation))),
};
let func = Func::new(move |xs| {
let xs = conv.forward(xs)?;
let xs = if let Some(bn) = &bn { xs.apply_t(bn, false)? } else { xs };
if leaky {
let temp = xs.clone();
Ok(temp.maximum(&(xs * 0.1)?)?)
} else {
Ok(xs)
}
});
Ok((filters, Bl::Layer(Box::new(func))))
}
/// The entry point for the program.
/// Demonstrates parsing a Darknet configuration file.
fn main() -> Result<()> {
println!("Parsing configuration and building Darknet...");
let darknet = parse_config("path/to/config.cfg")?; // Replace with your configuration file path.
println!("Parsed Darknet configuration: {:?}", darknet); // Print the parsed configuration.
Ok(())
}
This Rust code parses a Darknet configuration file, typically used to define neural networks like YOLO, and constructs a structured representation of the network. The Block
struct represents individual sections of the configuration, such as convolutional or YOLO layers, with their parameters stored as key-value pairs. The Darknet
struct aggregates these blocks and global parameters, forming the complete network structure. The Accumulator
helps manage and group parameters into blocks while parsing the file line by line, skipping comments and whitespace. Specialized functions like conv
create specific layers, such as convolutional layers, based on the configuration parameters. The code also defines an enum Bl
to represent different types of layers, like routes, shortcuts, and YOLO layers. The main
function demonstrates the process by parsing a sample configuration file and printing the resulting structure, providing a foundation for building and training neural networks based on Darknet configurations.
YOLOv8, or You Only Look Once version 8, is part of the well-established YOLO family of object detection models designed for real-time applications. The model's architecture builds on the success of its predecessors by incorporating modern techniques to improve both accuracy and speed. YOLOv8 retains the core principles of the YOLO design, focusing on efficiency without compromising detection performance. The architecture is typically broken down into three primary components: the Backbone, Neck, and Head, each responsible for different stages of the object detection pipeline.
The Backbone is the initial stage of the network, responsible for extracting features from the input image. In YOLOv8, the Backbone uses a modernized version of the DarkNet architecture or a similar lightweight feature extractor, optimized for performance and speed. The Backbone performs a series of convolutions that downsample the image, reducing its spatial dimensions while increasing the depth of the feature maps. This allows the network to capture important high-level semantic features while retaining sufficient fine-grained details. Additionally, the Backbone likely uses skip connections—a common deep learning technique that allows features from earlier layers to be passed directly to deeper layers, improving the preservation of important spatial information. The resulting feature maps provide the foundational understanding of the image, which is necessary for subsequent object detection tasks.
The Neck plays a crucial role in refining and combining the features extracted by the Backbone. In YOLOv8, the Neck typically utilizes techniques like Feature Pyramid Networks (FPN) and Path Aggregation Networks (PANet) to handle the challenge of detecting objects at multiple scales. FPNs work by creating feature pyramids, which combine both low-level, high-resolution features (which are good for detecting small objects) with high-level, semantically rich features (which are better for large objects). PANet further improves feature aggregation by strengthening both top-down and bottom-up pathways, leading to more efficient and accurate fusion of features. These enhanced features are then passed to the next stage of the network, where they are further refined before making predictions.
The Head is the final component in the YOLOv8 architecture and is responsible for generating the model's predictions, including bounding box coordinates, class labels, and confidence scores. The Head processes the refined features from the Neck through additional convolutional layers to make predictions about the locations and types of objects in the image. YOLOv8 uses anchor boxes—predefined bounding boxes with specific sizes and aspect ratios—on which the model bases its predictions. For each anchor box, the model predicts the coordinates of the bounding box (center x
, center y
, width w
, height h
), a confidence score that indicates the model's certainty about the presence of an object, and class probabilities that assign the object to a specific class, such as "car," "person," or "dog." Non-Maximum Suppression (NMS) is then applied to remove redundant boxes, keeping only the highest-confidence predictions for each object.
The final output of YOLOv8 consists of the predicted bounding boxes, class labels, and their associated confidence scores. This output is processed in such a way that it provides precise object localization and classification. The model is also optimized for speed and efficiency, making it suitable for real-time applications. YOLOv8 is capable of detecting objects across a wide range of sizes, from small objects like pedestrians to large objects like cars, thanks to the multi-scale feature fusion provided by the Backbone and Neck. Additionally, the architecture can be extended to tasks beyond basic object detection, such as pose estimation and keypoint detection, by predicting keypoints on human bodies or other objects, which further enhances its versatility.
Overall, YOLOv8 continues the evolution of the YOLO model family by refining the architecture and incorporating new advancements in deep learning. Its lightweight design ensures fast performance while maintaining high accuracy, making it an ideal solution for real-time object detection in a wide variety of applications. The modular nature of the architecture allows it to be adapted for specific tasks, such as standard object detection or more complex applications like human pose recognition.
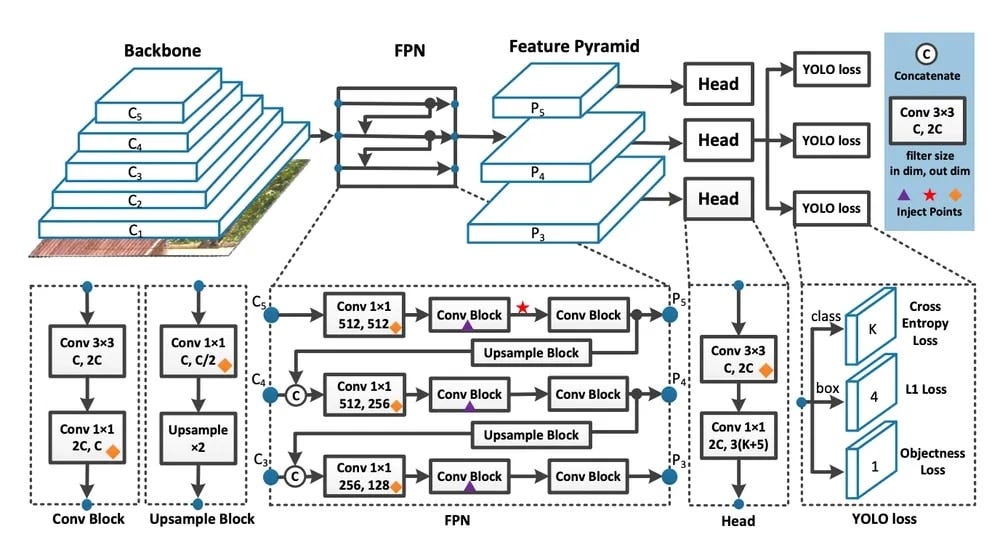
Figure 9: The complexity of YOLO v8 architecture model.
The code provides a framework for parsing and constructing neural network architectures based on Darknet configuration files, a format widely used for defining deep learning models like YOLO. It enables the creation of a structured representation of a neural network by interpreting configuration files, applying parameters to individual blocks (e.g., convolutional or YOLO layers), and assembling them into a cohesive model. Additionally, the code includes utilities for image manipulation, such as drawing bounding boxes, to support visualization tasks often required in object detection.
use candle_nn::{batch_norm, conv2d, conv2d_no_bias, Conv2dConfig, Func, Module, VarBuilder};
use image::{ImageBuffer}; // Removed DynamicImage since it wasn't used
use std::collections::BTreeMap; // For structured key-value parameter storage.
/// Represents a block in the Darknet configuration file.
/// Each block has a type (e.g., "convolutional", "yolo") and a set of parameters.
#[derive(Debug)]
struct Block {
block_type: String, // The type of block (e.g., "convolutional").
parameters: BTreeMap<String, String>, // Parameters for the block as key-value pairs.
}
impl Block {
/// Retrieves the value of a parameter by its key.
/// Returns an error if the key does not exist.
fn get(&self, key: &str) -> Result<&str, candle_core::Error> {
self.parameters
.get(key) // Try to get the key from the parameters.
.ok_or_else(|| candle_core::Error::Msg(format!("cannot find {} in {}", key, self.block_type))) // Error if not found.
.map(|x| x.as_str()) // Convert &String to &str.
}
}
/// Represents the entire Darknet configuration, which includes a series of blocks.
#[derive(Debug)]
pub struct Darknet {
blocks: Vec<Block>, // List of blocks parsed from the configuration file.
parameters: BTreeMap<String, String>, // Global parameters defined in the "net" block.
}
impl Darknet {
/// Retrieves the value of a global parameter by its key.
/// Returns an error if the key does not exist.
fn get(&self, key: &str) -> Result<&str, candle_core::Error> {
self.parameters
.get(key) // Try to get the key from global parameters.
.ok_or_else(|| candle_core::Error::Msg(format!("cannot find {} in net parameters", key))) // Error if not found.
.map(|x| x.as_str()) // Convert &String to &str.
}
}
/// Represents a layer or operation in the Darknet model.
enum Bl {
Layer(Box<dyn Module + Send + Sync>), // A standard layer.
Route(Vec<usize>), // A route layer combining outputs from other layers.
Shortcut(usize), // A shortcut layer (e.g., residual connection).
Yolo(usize, Vec<(usize, usize)>), // A YOLO detection layer.
}
/// Utility functions for drawing rectangles on images.
pub fn draw_rect(
img: &mut ImageBuffer<image::Rgb<u8>, Vec<u8>>,
x1: u32,
x2: u32,
y1: u32,
y2: u32,
) {
for x in x1..=x2 {
let pixel = img.get_pixel_mut(x, y1);
*pixel = image::Rgb([255, 0, 0]); // Draw top and bottom edges in red.
let pixel = img.get_pixel_mut(x, y2);
*pixel = image::Rgb([255, 0, 0]);
}
for y in y1..=y2 {
let pixel = img.get_pixel_mut(x1, y);
*pixel = image::Rgb([255, 0, 0]); // Draw left and right edges in red.
let pixel = img.get_pixel_mut(x2, y);
*pixel = image::Rgb([255, 0, 0]);
}
}
/// Model structure definitions for hyperparameters.
/// This struct is used to define scaling multipliers for the network.
#[derive(Debug)]
pub struct Multiples {
depth: f64, // Depth multiplier for network scaling.
width: f64, // Width multiplier for network scaling.
ratio: f64, // Additional scaling ratio.
}
impl Multiples {
/// Constructor for default scaling multipliers.
pub fn default() -> Self {
Multiples {
depth: 0.33, // Default depth multiplier.
width: 0.50, // Default width multiplier.
ratio: 1.0, // Default ratio for scaling.
}
}
/// Example usage of the fields to address the dead code warning.
pub fn print_values(&self) {
println!(
"Depth multiplier: {}, Width multiplier: {}, Ratio: {}",
self.depth, self.width, self.ratio
);
}
}
/// Builds a convolutional layer based on the configuration block.
fn conv(
vb: VarBuilder,
index: usize,
p: usize,
b: &Block,
) -> Result<(usize, Bl), candle_core::Error> {
let activation = b.get("activation")?;
let filters = b.get("filters")?.parse::<usize>()?;
let pad = b.get("pad")?.parse::<usize>()?;
let size = b.get("size")?.parse::<usize>()?;
let stride = b.get("stride")?.parse::<usize>()?;
let padding = if pad != 0 { (size - 1) / 2 } else { 0 };
let (bn, bias) = match b.parameters.get("batch_normalize") {
Some(p) if p.parse::<usize>()? != 0 => {
let bn = batch_norm(filters, 1e-5, vb.pp(format!("batch_norm_{index}")))?;
(Some(bn), false)
}
_ => (None, true),
};
let conv_cfg = Conv2dConfig {
stride,
padding,
groups: 1,
dilation: 1,
};
let conv = if bias {
conv2d(p, filters, size, conv_cfg, vb.pp(format!("conv_{index}")))?
} else {
conv2d_no_bias(p, filters, size, conv_cfg, vb.pp(format!("conv_{index}")))?
};
let leaky = match activation {
"leaky" => true,
"linear" => false,
_ => return Err(candle_core::Error::Msg(format!("unsupported activation function: {}", activation))),
};
let func = Func::new(move |xs| {
let mut xs = conv.forward(xs)?;
// Apply batch normalization if it exists.
if let Some(bn) = &bn {
xs = xs.apply_t(bn, false)?;
}
// Modify `xs` based on activation type without moving it.
if leaky {
let xs_ref = &xs;
let result = xs_ref.maximum(&(xs_ref * 0.1)?)?;
Ok(result)
} else {
Ok(xs)
}
});
Ok((filters, Bl::Layer(Box::new(func))))
}
/// Parses a Darknet configuration file and constructs a `Darknet` object.
pub fn parse_config<T: AsRef<std::path::Path>>(path: T) -> Result<Darknet, candle_core::Error> {
use std::fs::File;
use std::io::{BufRead, BufReader};
let file = File::open(path.as_ref())?; // Open the file.
let mut acc = Accumulator::new(); // Create a new accumulator.
for line in BufReader::new(file).lines() {
let line = line?;
if line.is_empty() || line.starts_with('#') {
continue; // Skip empty lines and comments.
}
let line = line.trim();
if line.starts_with('[') {
// A new block starts.
if !line.ends_with(']') {
return Err(candle_core::Error::Msg(format!("line does not end with ']' {line}")));
}
acc.finish_block(); // Finalize the previous block.
acc.block_type = Some(line[1..line.len() - 1].to_string()); // Set the new block type.
} else {
// Parse key-value pairs.
let key_value: Vec<&str> = line.splitn(2, '=').collect();
if key_value.len() != 2 {
return Err(candle_core::Error::Msg(format!("missing equal {line}")));
}
let prev = acc.parameters.insert(
key_value[0].trim().to_owned(),
key_value[1].trim().to_owned(),
);
if prev.is_some() {
return Err(candle_core::Error::Msg(format!("multiple values for key {}", line)));
}
}
}
acc.finish_block(); // Finalize the last block.
Ok(acc.net) // Return the parsed Darknet configuration.
}
/// Represents a block accumulator while parsing the configuration.
struct Accumulator {
block_type: Option<String>, // The type of the current block being parsed.
parameters: BTreeMap<String, String>, // Parameters for the current block.
net: Darknet, // The resulting Darknet model.
}
impl Accumulator {
fn new() -> Self {
Self {
block_type: None,
parameters: BTreeMap::new(),
net: Darknet {
blocks: Vec::new(),
parameters: BTreeMap::new(),
},
}
}
fn finish_block(&mut self) {
if let Some(block_type) = self.block_type.take() {
let block = Block {
block_type,
parameters: std::mem::take(&mut self.parameters),
};
self.net.blocks.push(block);
}
}
}
/// Main function to demonstrate the model is ready for use.
fn main() {
// Use the Multiples struct to avoid warnings.
let multiples = Multiples::default();
multiples.print_values();
println!("Darknet model loaded and ready for inference!");
}
The code begins by defining the Block
and Darknet
structures to parse and represent the network's configuration. The parse_config
function processes the Darknet configuration file, identifying blocks and their parameters, which are stored in a structured format. Individual layers are constructed dynamically based on their type using the conv
function, which applies transformations like convolution, batch normalization, and activation functions (e.g., leaky ReLU). The Accumulator
ensures seamless parsing by grouping parameters into blocks. A utility function, draw_rect
, is included for image visualization by rendering bounding boxes. The Multiples
struct allows for configurable scaling of network depth, width, and ratios, demonstrating flexibility for creating custom model variants.
To deepen your understanding of YOLO (You Only Look Once) models and utilize the low-code tools offered by Ultralytics, it’s essential to adopt a systematic approach that blends theoretical learning, practical experimentation, and exploration of advanced tools. YOLO models are widely recognized for their real-time object detection capabilities, achieved through a single-stage detection approach that combines speed and accuracy. Begin by studying the evolution of YOLO, starting with YOLOv1, which introduced grid-based predictions, to YOLOv3’s anchor-based design. Progress further to YOLOv4 and YOLOv5, which emphasize optimizations like CSPDarknet and Mosaic augmentation. Finally, explore YOLOv8, the most recent iteration by Ultralytics, which simplifies training, enhances performance, and integrates user-friendly tools.
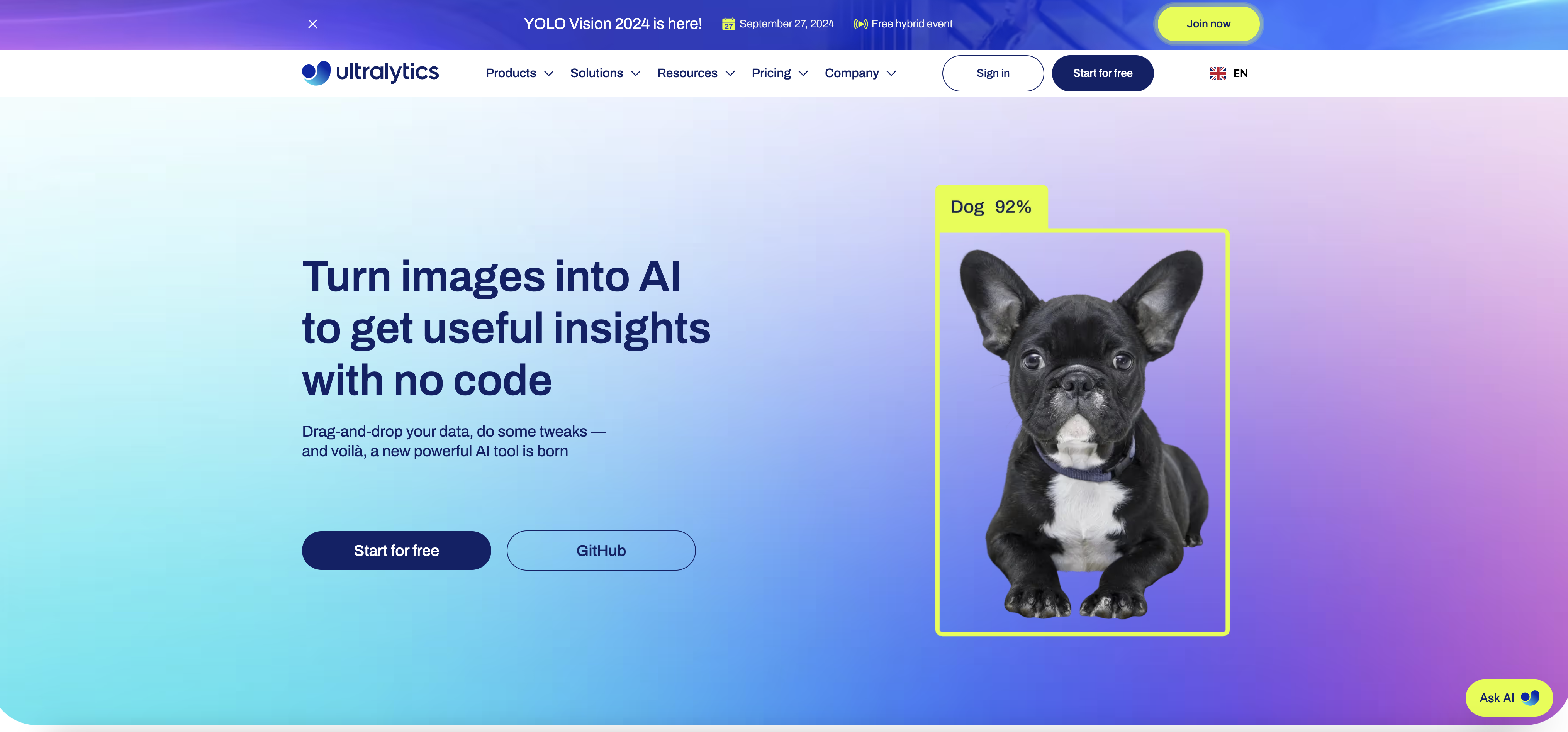
Figure 10: Ultralytics platform powered by YOLO models.
Understanding the mathematical foundations is crucial to mastering YOLO models. Study the principles of bounding box regression, confidence scoring, and techniques like Intersection over Union (IoU) and non-maximum suppression (NMS), which refine predictions by suppressing overlapping detections. Loss functions in YOLO, including objectness loss, classification loss, and localization loss, are also key components. Familiarize yourself with the challenges these models address, such as balancing real-time detection and precision, as well as their applications across industries like autonomous driving, healthcare, and security.
Ultralytics has developed a user-friendly ecosystem around YOLO models, which simplifies complex tasks such as model training, inference, and export. By installing the Ultralytics Python package, you gain access to pre-trained YOLOv8 models and the ability to train custom models with minimal code. Using commands for training, prediction, and exporting, you can quickly build robust object detection systems. This low-code approach makes it easier for beginners and professionals to get started, without requiring extensive expertise in deep learning.
Practical experimentation is the cornerstone of learning. Start with well-annotated datasets like COCO or Pascal VOC or use tools like LabelImg or Roboflow to label custom datasets. Train YOLO models using the Ultralytics platform, fine-tuning hyperparameters and leveraging pre-trained weights for transfer learning. Evaluate your model’s performance using metrics such as mean Average Precision (mAP) and precision-recall analysis. Experimenting with different datasets and model configurations will provide hands-on experience in optimizing detection systems.
As you advance, delve into customizing YOLO models for specific use cases. Modify the model architecture to accommodate unique tasks, such as multi-class detection or regression. Integrate YOLO with other low-code tools like Roboflow for data preprocessing and Edge Impulse for edge deployments. Explore YOLOv8-specific enhancements, such as its faster non-maximum suppression and flexible architecture, to stay at the forefront of innovation.
Finally, engage with the Ultralytics and AI community to stay updated on the latest advancements. Resources like Ultralytics’ GitHub repository and documentation are excellent starting points. Joining forums, participating in discussions on platforms like Discord or Stack Overflow, and enrolling in online courses focused on YOLO and object detection will further enhance your skills. Combining this knowledge with real-world applications, such as deploying YOLO models on edge devices or integrating them into cloud-based APIs, will solidify your expertise in this transformative technology.
6.8. Conclusion
Chapter 6 equips you with the knowledge and tools to implement and optimize modern CNN architectures using Rust. By understanding both the fundamental concepts and advanced techniques, you are well-prepared to build powerful, efficient, and scalable CNN models that take full advantage of Rust's performance capabilities.
6.8.1. Further Learning with GenAI
These prompts are designed to challenge your understanding of modern CNN architectures and their implementation in Rust. Each prompt encourages exploration of advanced concepts, architectural innovations, and practical challenges in building and training state-of-the-art CNNs.
Analyze the evolution of CNN architectures from VGG to EfficientNet, MobileNet, and YOLO. How have innovations such as depth, residual connections, compound scaling, efficient depthwise separable convolutions, and real-time object detection influenced the design and performance of modern CNNs, and how can these concepts be effectively implemented in Rust?
Discuss the architectural simplicity and depth of VGG networks. How does VGG's use of small (3x3) filters contribute to its performance, and what are the trade-offs between simplicity and computational efficiency when implementing VGG in Rust? Contrast this simplicity with the lightweight, efficient design of MobileNet, which employs depthwise separable convolutions for reduced computational cost.
Examine the role of residual connections in ResNet. How do these connections mitigate the vanishing gradient problem in very deep networks, and how can they be implemented in Rust to ensure stable and efficient training of large-scale models? Additionally, explore how YOLO employs residual connections in its backbone to maintain efficiency and performance.
Explore the concept of multi-scale feature extraction in Inception networks. How do Inception modules enhance a model's ability to capture complex patterns, and what are the challenges of implementing multi-scale architectures in Rust using tch-rs or burn? Compare this approach to YOLO's real-time multi-scale object detection capabilities.
Investigate the impact of dense connectivity in DenseNet. How does DenseNet's approach to feature reuse improve model performance with fewer parameters, and what are the key considerations when implementing dense blocks in Rust? Additionally, examine how YOLO integrates feature pyramids for hierarchical detection.
Discuss the principles of compound scaling in EfficientNet and the lightweight scaling strategies of MobileNet. How does EfficientNet balance depth, width, and resolution to achieve high performance with minimal computational cost? How does MobileNet leverage depthwise separable convolutions for similar goals? What are the best practices for implementing these scaling strategies in Rust?
Evaluate the scalability of modern CNN architectures like ResNet, DenseNet, MobileNet, and YOLO. How can Rust be used to scale these architectures across multiple devices or distributed systems, and what are the trade-offs in terms of synchronization and computational efficiency? Discuss YOLO’s real-time capabilities in the context of scalability.
Analyze the process of training very deep CNNs, such as ResNet-152, DenseNet-201, and YOLO's larger variants. What are the challenges in managing memory and computational resources in Rust, and how can advanced techniques like mixed precision training be applied to optimize performance while supporting real-time constraints?
Explore the role of neural architecture search (NAS) in discovering optimal CNN configurations. How can Rust be leveraged to implement NAS algorithms, and what are the potential benefits of using NAS to optimize architectures like EfficientNet, MobileNet, and YOLO for specific tasks?
Examine the trade-offs between accuracy and computational efficiency in modern CNNs. How can Rust be used to implement and compare architectures like ResNet, EfficientNet, MobileNet, and YOLO, and what strategies can be employed to balance model performance with resource constraints in real-time applications?
Discuss the importance of modularity in modern CNN architectures. How can Rust's type system and modular design capabilities be leveraged to create flexible and reusable CNN components, enabling the integration of features from YOLO, MobileNet, and EfficientNet for easy experimentation and adaptation?
Investigate the integration of modern CNN architectures with pre-trained models. How can Rust be used to fine-tune pre-trained models like ResNet, EfficientNet, MobileNet, or YOLO for specific tasks, and what are the challenges in adapting these models to new domains and datasets?
Analyze the role of attention mechanisms in enhancing CNN performance. How can attention modules be incorporated into modern CNN architectures in Rust, and what are the potential benefits of combining attention with traditional convolutional layers and YOLO’s detection pipelines?
Explore the implementation of custom CNN architectures in Rust. How can Rust be used to design and train novel CNN models that incorporate elements from multiple modern architectures, such as combining residual connections, depthwise convolutions, dense blocks, or YOLO’s detection strategies?
Discuss the impact of data augmentation on the training of modern CNNs. How can Rust be utilized to implement advanced data augmentation techniques, and what are the best practices for ensuring that augmentation improves model robustness without introducing artifacts? Highlight its relevance to YOLO’s detection tasks.
Examine the role of transfer learning in modern CNN architectures. How can Rust-based implementations of models like YOLO and MobileNet be fine-tuned for new tasks using transfer learning, and what are the key considerations in preserving the accuracy of the original model while adapting to new data?
Analyze the debugging and profiling tools available in Rust for modern CNN architectures. How can these tools be used to identify and resolve performance bottlenecks in complex CNN models like YOLO or EfficientNet, ensuring that both training and inference are optimized?
Investigate the use of GPUs and parallel processing in accelerating the training of modern CNNs in Rust. How can Rust's concurrency and parallelism features be leveraged to enhance the performance of deep learning models on modern hardware, particularly for real-time YOLO implementations?
Explore the role of hyperparameter tuning in optimizing modern CNN architectures. How can Rust be used to automate the tuning process for models like YOLO, MobileNet, and EfficientNet, and what are the most critical hyperparameters influencing their training and performance?
Discuss the future directions of CNN research and how Rust can contribute to advancements in deep learning. What emerging trends and technologies in CNN architecture, such as self-supervised learning, capsule networks, or improved versions of YOLO, can be supported by Rust's unique features for high-performance and modular design?
By engaging with these comprehensive and challenging questions, you will gain the insights and skills necessary to build, optimize, and innovate in the field of deep learning. Let these prompts guide your exploration and inspire you to push the boundaries of what is possible with modern CNNs and Rust.
6.8.2. Hands On Practices
These exercises are designed to provide in-depth, practical experience with the implementation and optimization of modern CNN architectures in Rust. They challenge you to apply advanced techniques and develop a strong understanding of cutting-edge CNN models through hands-on coding, experimentation, and analysis.
Exercise 6.1: Implementing and Fine-Tuning a VGG Network in Rust
Task: Implement the VGG architecture in Rust using the
tch-rs
crate. Train the model on a dataset like CIFAR-10, and fine-tune the network to achieve optimal performance. Focus on the impact of depth and small filters on model accuracy and training efficiency.Challenge: Experiment with different VGG variants by adjusting the number of layers and filter sizes. Compare the performance of your models, and analyze the trade-offs between simplicity, accuracy, and computational cost.
Exercise 6.2: Building and Training a ResNet Model with Residual Connections
Task: Implement the ResNet architecture in Rust, focusing on the correct implementation of residual connections. Train the model on a large dataset like ImageNet, and analyze the impact of residual connections on training stability and accuracy.
Challenge: Experiment with different ResNet depths (e.g., ResNet-18, ResNet-50, ResNet-152) and evaluate the trade-offs between model complexity, training time, and accuracy. Implement techniques like mixed precision training to optimize resource usage.
Exercise 6.3: Designing and Implementing Custom Inception Modules
Task: Create custom Inception modules in Rust by combining different convolutional paths within a single layer. Implement these modules in a CNN architecture, and train the model on a dataset like ImageNet to evaluate its ability to capture multi-scale features.
Challenge: Experiment with different configurations of Inception modules, such as varying the number of paths and types of operations (e.g., convolutions, pooling). Compare the performance of your custom modules with standard Inception models.
Exercise 6.4: Implementing DenseNet and Exploring Feature Reuse
Task: Implement the DenseNet architecture in Rust, focusing on the dense connectivity and feature reuse across layers. Train the model on a dataset like CIFAR-10, and analyze the impact of dense blocks on model accuracy and parameter efficiency.
Challenge: Experiment with different growth rates and block configurations to optimize model performance. Compare the parameter efficiency and accuracy of DenseNet with other modern CNN architectures like ResNet and VGG.
Exercise 6.5: Implementing EfficientNet and Exploring Compound Scaling
Task: Implement the EfficientNet architecture in Rust using the
tch-rs
crate. Train the model on a complex dataset like ImageNet, focusing on the compound scaling method to balance depth, width, and resolution.Challenge: Experiment with different scaling factors to optimize model performance while minimizing computational cost. Compare the efficiency and accuracy of EfficientNet with other modern CNN architectures, and analyze the benefits of compound scaling.
By completing these challenges, you will gain hands-on experience and develop a deep understanding of the complexities involved in building state-of-the-art CNN models, preparing you for advanced work in deep learning and AI.
Comments